spring request重定向携带参数
时间: 2023-09-23 19:12:12 浏览: 110
在Spring中,可以通过使用RedirectAttributes来实现重定向时携带参数。它可以在重定向时将参数添加到URL中,而不是将它们作为简单的查询参数。
以下是一个简单的示例:
```
@PostMapping("/submit-form")
public String submitForm(@ModelAttribute("formData") FormData formData, RedirectAttributes redirectAttributes) {
// 处理表单数据
redirectAttributes.addFlashAttribute("message", "表单已成功提交!");
return "redirect:/thank-you";
}
@GetMapping("/thank-you")
public String thankYou(@ModelAttribute("message") String message) {
// 显示感谢页面
return "thank-you";
}
```
在上面的示例中,当表单提交成功时,会将一条消息添加到RedirectAttributes中,然后重定向到“/thank-you”URL。在“/thank-you”控制器方法中,消息将作为ModelAttribute注释的参数自动注入,并在感谢页面中显示出来。
注意:使用addFlashAttribute方法可以确保参数只会在重定向后一次请求中使用,而不是在多个请求之间共享。
相关问题
spring boot重定向携带参数和请求头
在Spring Boot中,可以使用`RedirectAttributes`来将参数传递给重定向后的页面,使用`HttpHeaders`来设置请求头。
下面是一个示例代码:
```java
@GetMapping("/redirectWithParams")
public String redirectWithParams(RedirectAttributes attributes) {
attributes.addAttribute("param1", "value1");
attributes.addAttribute("param2", "value2");
return "redirect:/destination";
}
@GetMapping("/redirectWithHeaders")
public ResponseEntity<Object> redirectWithHeaders() {
HttpHeaders headers = new HttpHeaders();
headers.set("Custom-Header", "value");
return ResponseEntity.status(HttpStatus.FOUND)
.headers(headers)
.location(URI.create("/destination"))
.build();
}
@GetMapping("/destination")
public String destination(@RequestParam String param1, @RequestParam String param2) {
// do something with the parameters
return "destination";
}
```
在`redirectWithParams`方法中,我们将参数添加到`RedirectAttributes`中,并返回重定向到目标页面的字符串。在目标页面中,我们可以使用`@RequestParam`注解来获取参数值。
在`redirectWithHeaders`方法中,我们创建一个`HttpHeaders`对象并设置自定义请求头。然后,我们使用`ResponseEntity`来创建一个重定向响应,并将`HttpHeaders`对象和目标URL传递给`location`方法。
请注意,`redirectWithHeaders`方法返回`ResponseEntity`而不是字符串,因为我们需要设置响应状态码和头信息。
spring boot重定向请求加入认证参数
在Spring Boot应用中,如果你想要在重定向(Redirect)请求时添加认证相关的参数,比如JWT令牌,通常会在HTTP响应头设置`Location`字段,同时将认证信息作为查询参数传递。以下是步骤:
1. **创建JWT Token**:首先确保你有一个生成JWT token的机制,例如使用Spring Security的JwtEncoder或者自定义的一个工具类。
```java
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.context.SecurityContextHolder;
import com.auth0.jwt.JWT;
import com.auth0.jwt.algorithms.Algorithm;
public String generateJwtToken(String username) {
// 使用用户名和密钥生成JWT
return JWT.create()
.withSubject(username)
.sign(Algorithm.HMAC256("your-secret-key"));
}
```
2. **登录成功后的重定向**:当用户通过身份验证并登录成功后,在`SecurityController`或其他相关控制器中处理登录,获取到JWT,然后将其添加到重定向URL的查询参数中:
```java
@GetMapping("/login")
public RedirectView login(@RequestParam String username, String password) {
// 验证用户名密码...
if (isValidUser()) {
UsernamePasswordAuthenticationToken auth = new UsernamePasswordAuthenticationToken(username, password);
SecurityContextHolder.getContext().setAuthentication(auth);
// 添加JWT到查询参数
String jwt = generateJwtToken(username);
String redirectUrl = "http://example.com/someprotectedpage?jwt=" + jwt;
return new RedirectView(redirectUrl, true); // 设置true表示永久重定向
}
// 登录失败处理...
}
```
3. **保护资源的拦截器**:在需要保护的控制器方法上,可以配置一个JWT过滤器(如JwtRequestFilter)来检查查询参数中的JWT是否有效:
```java
@Component
public class JwtRequestFilter extends OncePerRequestFilter {
@Autowired
private JwtHelper jwtHelper;
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain)
throws ServletException, IOException {
String jwt = request.getParameter("jwt");
if (jwt != null && validateJwt(jwt)) {
// JWT有效,继续执行后续操作
chain.doFilter(request, response);
} else {
// JWT无效,处理未授权或返回错误页面
response.sendRedirect("/unauthorized"); // 或者返回401状态码
}
}
private boolean validateJwt(String jwt) {
try {
JWT.verify(jwt, Algorithm.HMAC256("your-secret-key"));
return true;
} catch (Exception e) {
return false;
}
}
}
```
阅读全文
相关推荐
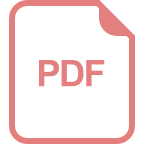
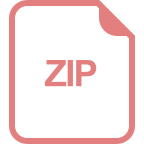
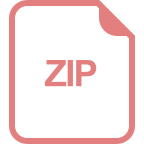













