用python打开csv并用一段数据画图
时间: 2023-04-24 08:05:43 浏览: 191
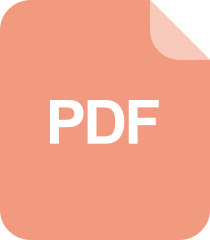
Python对CSV文件数据进行可视化

可以使用Python的pandas库来打开csv文件,并使用matplotlib库来绘制图表。
以下是一个示例代码,假设我们有一个名为data.csv的文件,其中包含以下数据:
```
Year,Population
1950,2.5
1960,3.0
1970,3.7
1980,4.4
1990,5.3
2000,6.1
2010,6.9
```
我们可以使用以下代码来打开csv文件并绘制折线图:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 读取csv文件
data = pd.read_csv('data.csv')
# 绘制折线图
plt.plot(data['Year'], data['Population'])
plt.xlabel('Year')
plt.ylabel('Population (billions)')
plt.title('World Population')
plt.show()
```
运行代码后,将会显示一个折线图,其中x轴表示年份,y轴表示人口数量。
阅读全文
相关推荐
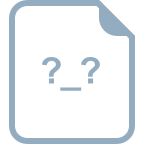
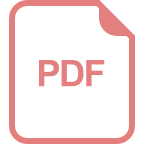
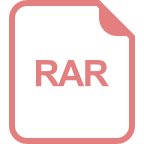
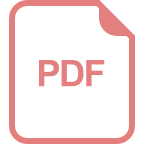
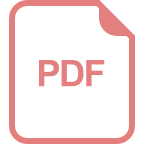
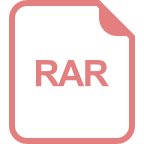
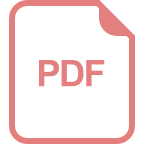
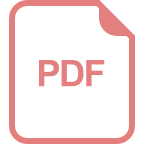
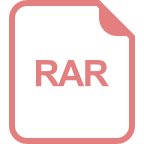
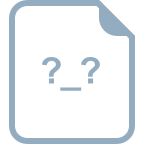
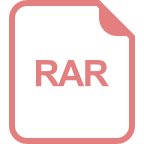
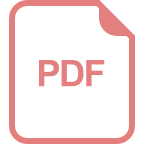