import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.http.HttpMethod; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.builders.WebSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder; import org.springframework.security.crypto.password.PasswordEncoder; @Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Autowired private JwtTokenProvider jwtTokenProvider; @Override protected void configure(HttpSecurity http) throws Exception { http.csrf().disable().authorizeRequests() .antMatchers("/api/**").authenticated() .and() .apply(new JwtConfigurer(jwtTokenProvider)); } @Override public void configure(WebSecurity web) throws Exception { web.ignoring().antMatchers(HttpMethod.OPTIONS, "/**"); } @Bean public PasswordEncoder passwordEncoder() { return new BCryptPasswordEncoder(12); } }报错,帮我修改一下
时间: 2023-12-14 21:36:13 浏览: 31
您需要引入以下的包:
```java
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.http.HttpMethod;
import org.springframework.context.annotation.Bean;
import org.springframework.security.config.annotation.web.builders.WebSecurity;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.beans.factory.annotation.Autowired;
```
然后,您需要将`SecurityConfig`类继承自`WebSecurityConfigurerAdapter`类。在类上添加`@Configuration`和`@EnableWebSecurity`注解。
接下来,您需要重写`configure(HttpSecurity http)`方法。您可以使用`.csrf().disable()`禁用CSRF保护,`.authorizeRequests().antMatchers("/api/**").authenticated()`配置只有经过身份验证的用户才能访问您的API。最后,您需要使用`apply()`方法将您的`JwtConfigurer`类应用于HttpSecurity。
然后,您需要重写`configure(WebSecurity web)`方法并忽略OPTIONS请求。
最后,您需要创建一个名为`passwordEncoder()`的方法,该方法返回一个`BCryptPasswordEncoder`实例。这个实例将用于对密码进行加密。
以下是修改后的代码:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private JwtTokenProvider jwtTokenProvider;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable().authorizeRequests()
.antMatchers("/api/**").authenticated()
.and()
.apply(new JwtConfigurer(jwtTokenProvider));
}
@Override
public void configure(WebSecurity web) throws Exception {
web.ignoring().antMatchers(HttpMethod.OPTIONS, "/**");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder(12);
}
}
```
相关推荐
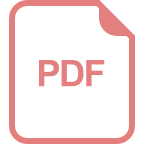
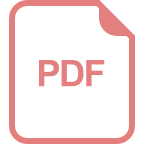
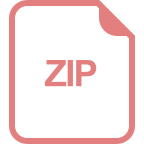














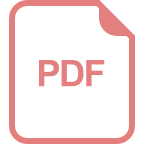
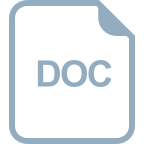