帮我用c语言写一份粒子光线生长树的代码
时间: 2023-06-12 21:03:11 浏览: 165
粒子光线生长树(Particle Light Tree)是一种基于粒子系统的树形结构生成算法。下面是一个简单的粒子光线生长树的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_PARTICLES 10000
#define MAX_CHILDREN 10
#define MAX_LEVELS 10
typedef struct {
float x, y, z;
} Vector3;
typedef struct Particle {
Vector3 position;
int num_children;
struct Particle* children[MAX_CHILDREN];
} Particle;
Particle particles[MAX_PARTICLES];
int num_particles = 0;
/**
* 生成一个随机向量
*/
Vector3 random_vector() {
Vector3 v;
v.x = (float)rand() / RAND_MAX;
v.y = (float)rand() / RAND_MAX;
v.z = (float)rand() / RAND_MAX;
return v;
}
/**
* 生成一个新的粒子
*/
Particle* new_particle(Vector3 position) {
Particle* p = &particles[num_particles++];
p->position = position;
p->num_children = 0;
return p;
}
/**
* 在指定粒子上添加一个子粒子
*/
void add_child(Particle* parent, Particle* child) {
if (parent->num_children < MAX_CHILDREN) {
parent->children[parent->num_children++] = child;
}
}
/**
* 递归生长粒子光线生长树
*/
void grow_tree(Particle* parent, int level) {
if (level >= MAX_LEVELS) {
return;
}
for (int i = 0; i < 3; i++) {
Particle* child = new_particle(random_vector());
add_child(parent, child);
grow_tree(child, level + 1);
}
}
/**
* 打印粒子光线生长树的结构
*/
void print_tree(Particle* parent, int level) {
for (int i = 0; i < level; i++) {
printf(" ");
}
printf("%.2f, %.2f, %.2f\n", parent->position.x, parent->position.y, parent->position.z);
for (int i = 0; i < parent->num_children; i++) {
print_tree(parent->children[i], level + 1);
}
}
int main() {
srand(time(NULL));
Particle* root = new_particle(random_vector());
grow_tree(root, 0);
print_tree(root, 0);
return 0;
}
```
这个实现是比较简单的,仅仅是生成了一棵随机的粒子光线生长树,并将其结构打印出来。你可以根据自己的需求对其进行修改和扩展。
相关推荐
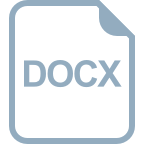
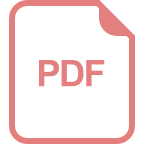














