Django数据可视化项目源码示例
时间: 2023-09-23 10:11:31 浏览: 58
以下是一个基于Django的数据可视化项目的源码示例,该项目使用了Python的matplotlib库和pandas库来生成图表和处理数据。
在该示例项目中,我们将使用一个名为"students"的数据表,其中包含每个学生的姓名、年龄和成绩。我们将使用matplotlib库来绘制学生成绩的分布图和散点图。
首先,我们需要在Django中创建一个app,并在其中定义一个view函数来处理数据和渲染模板。以下是一个简单的视图函数示例:
```python
# views.py
from django.shortcuts import render
import pandas as pd
import matplotlib.pyplot as plt
def student_performance(request):
# 从数据库中获取数据
data = Student.objects.all().values('name', 'age', 'score')
# 转换为pandas DataFrame
df = pd.DataFrame.from_records(data)
# 统计成绩分布
bins = [0, 60, 70, 80, 90, 100]
groups = df.groupby(pd.cut(df['score'], bins=bins)).size()
# 生成分布图
fig, ax = plt.subplots()
groups.plot.bar(ax=ax)
ax.set_xlabel('Score')
ax.set_ylabel('Count')
ax.set_title('Distribution of Scores')
# 生成散点图
fig2, ax2 = plt.subplots()
df.plot.scatter(x='age', y='score', ax=ax2)
ax2.set_xlabel('Age')
ax2.set_ylabel('Score')
ax2.set_title('Scatter Plot of Age vs. Score')
# 将图表渲染到模板中
context = {
'scores': fig.canvas.to_html(),
'scatter': fig2.canvas.to_html(),
}
return render(request, 'student_performance.html', context)
```
在该视图函数中,我们首先从我们的数据库中获取了学生的数据,并将其转换为pandas DataFrame以便于处理。然后,我们使用pandas库来计算成绩分布,并使用matplotlib库来生成分布图和散点图。
最后,我们将图表渲染到模板中,以便在浏览器中显示。以下是一个简单的模板示例:
```html
<!-- student_performance.html -->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Student Performance</title>
</head>
<body>
<h1>Student Performance</h1>
<div>{{ scores|safe }}</div>
<div>{{ scatter|safe }}</div>
</body>
</html>
```
在该模板中,我们使用Django的模板语言来渲染图表,并使用safe过滤器来确保图表中的HTML代码不被转义。
最后,我们需要将该视图函数添加到Django中的URL配置中:
```python
# urls.py
from django.urls import path
from .views import student_performance
urlpatterns = [
path('student_performance/', student_performance, name='student_performance'),
]
```
现在,我们可以在浏览器中访问/student_performance/路径来查看我们生成的图表。
相关推荐
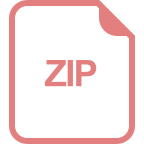
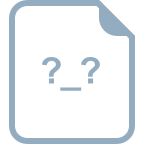
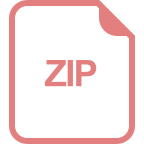
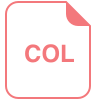
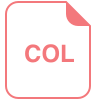
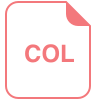
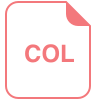
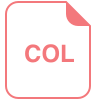









