javasprict用三种不同方式定义学生对象、姓名、学号、性别、年龄、学校、专业、班级年级,可以上课、写作业、做实验
时间: 2023-12-25 19:04:39 浏览: 162
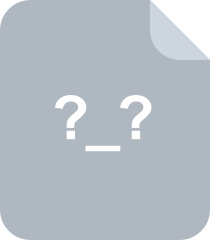
Java编写一个学生信息管理系统,实现学生信息包括:学号、姓名、性别、年龄、班级等信息
以下是三种不同方式定义学生对象的示例:
1. 使用对象字面量方式定义学生对象:
```javascript
var student1 = {
姓名: "张三",
学号: "20210001",
性别: "男",
年龄: 18,
学校: "ABC大学",
专业: "计算机科学",
班级年级: "2021级",
上课: function() {
console.log("上课中...");
},
写作业: function() {
console.log("写作业中...");
},
做实验: function() {
console.log("做实验中...");
}
};
```
2. 使用构造函数方式定义学生对象:
```javascript
function Student(姓名, 学号, 性别, 年龄, 学校, 专业, 班级年级) {
this.姓名 = 姓名;
this.学号 = 学号;
this.性别 = 性别;
this.年龄 = 年龄;
this.学校 = 学校;
this.专业 = 专业;
this.班级年级 = 班级年级;
this.上课 = function() {
console.log("上课中...");
};
this.写作业 = function() {
console.log("写作业中...");
};
this.做实验 = function() {
console.log("做实验中...");
};
}
var student2 = new Student("李四", "20210002", "女", 19, "DEF大学", "化学", "2021级");
```
3. 使用类和构造函数方式定义学生对象(ES6及以上版本):
```javascript
class Student {
constructor(姓名, 学号, 性别, 年龄, 学校, 专业, 班级年级) {
this.姓名 = 姓名;
this.学号 = 学号;
this.性别 = 性别;
this.年龄 = 年龄;
this.学校 = 学校;
this.专业 = 专业;
this.班级年级 = 班级年级;
}
上课() {
console.log("上课中...");
}
写作业() {
console.log("写作业中...");
}
做实验() {
console.log("做实验中...");
}
}
var student3 = new Student("王五", "20210003", "男", 20, "GHI大学", "物理", "2021级");
```
阅读全文
相关推荐
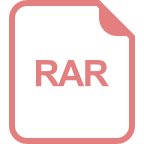
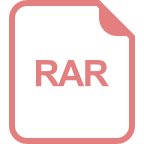



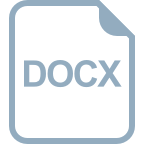
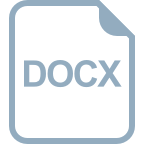
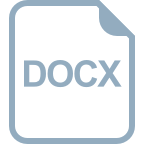
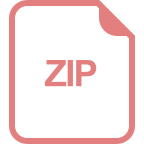