python 如何计算两个列表相同元素有多少个
时间: 2023-06-05 12:04:19 浏览: 1307
两个列表相同元素的数量可以使用 set() 函数将列表转换为集合类型,然后使用 & 运算符取出交集元素,最后使用 len() 函数计算交集元素的数量。代码如下:
```python
list1 = [1, 2, 3, 4, 5]
list2 = [3, 4, 5, 6, 7]
set1 = set(list1)
set2 = set(list2)
same_items = set1 & set2
num_same_items = len(same_items)
print(num_same_items)
```
输出结果为:3
相关问题
python获取两个列表相同元素
在Python中,你可以使用集合(set)或者并集运算符(`|`)以及相交运算符(`&`)来获取两个列表中的相同元素。以下是两种常见的方法:
1. **使用集合(Set)**:
首先将两个列表转换为集合,然后找出它们的交集,交集中的元素就是两个列表相同的元素。
```python
list1 = [1, 2, 3, 4]
list2 = [3, 4, 5, 6]
# 转换为集合
set1 = set(list1)
set2 = set(list2)
# 找出相同元素
common_elements = list(set1 & set2)
```
2. **使用并集运算符(`|`)**:
利用并集运算符可以合并两个列表,然后从结果中移除第一个列表,剩下的就是相同的元素。
```python
list1 = [1, 2, 3, 4]
list2 = [3, 4, 5, 6]
# 合并两个列表
merged_list = list1 + list2
# 删除第一个列表的元素
common_elements = [element for element in merged_list if element not in list1]
```
python 判断两个列表有多少相同元素
在 Python 中,判断两个列表中有多少相同元素可以使用集合(set)数据结构来完成。集合是一种无序且不包含重复元素的数据结构。你可以将两个列表转化为集合,然后求它们的交集,最后得到的就是相同的元素个数。以下是步骤:
```python
def count_common_elements(list1, list2):
set1 = set(list1)
set2 = set(list2)
common_elements = set1 & set2 # 使用 "&" 运算符获取交集
return len(common_elements)
list1 = [1, 2, 3, 4, 5]
list2 = [4, 5, 6, 7, 8]
print(count_common_elements(list1, list2))
```
如果你需要返回相同元素的具体列表,可以稍微修改一下函数:
```python
def find_common_elements(list1, list2):
set1 = set(list1)
set2 = set(list2)
common_elements = set1 & set2
return list(common_elements)
# 示例
common_list = find_common_elements(list1, list2)
print("Common elements:", common_list)
```
阅读全文
相关推荐
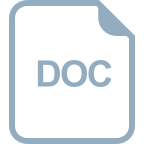
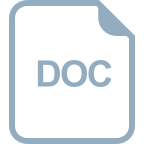
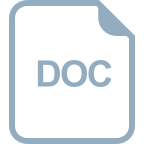
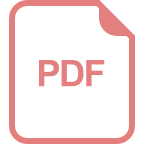
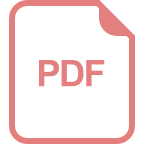
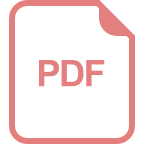










