X11 openGL 环境初始化
时间: 2023-11-30 15:01:49 浏览: 27
要初始化 X11 openGL 环境,你需要完成以下几个步骤:
1. 创建一个 X11 窗口,并将其与一个 OpenGL 上下文关联起来。
2. 加载所需的 OpenGL 扩展库。
3. 为 OpenGL 上下文设置像素格式。
4. 激活 OpenGL 上下文。
具体实现方式可以参考一些开源的 X11 OpenGL 应用程序,例如 Mesa3D、FreeGLUT 等。另外,也可以使用一些现成的图形库,例如 GLFW、SDL 等,它们提供了简便的接口来初始化和管理 OpenGL 环境。
相关问题
X11 openGL 环境初始化 代码示例
以下是使用 X11 和 OpenGL 初始化一个窗口的示例代码:
```c++
#include <X11/Xlib.h>
#include <GL/gl.h>
#include <GL/glx.h>
Display *display;
Window window;
GLXContext glContext;
int main()
{
// 1. 初始化 X11 窗口
display = XOpenDisplay(NULL);
if (display == NULL) {
printf("Error: cannot connect to X server\n");
return 1;
}
int screen = DefaultScreen(display);
Window rootWindow = RootWindow(display, screen);
int visualAttributes[] = {
GLX_RGBA,
GLX_DEPTH_SIZE, 24,
GLX_DOUBLEBUFFER,
None
};
XVisualInfo *visual = glXChooseVisual(display, screen, visualAttributes);
if (visual == NULL) {
printf("Error: cannot find suitable visual\n");
return 1;
}
XSetWindowAttributes windowAttributes;
windowAttributes.colormap = XCreateColormap(display, rootWindow, visual->visual, AllocNone);
windowAttributes.event_mask = ExposureMask | KeyPressMask;
window = XCreateWindow(display, rootWindow, 0, 0, 640, 480, 0, visual->depth, InputOutput, visual->visual, CWColormap | CWEventMask, &windowAttributes);
XMapWindow(display, window);
// 2. 加载所需的 OpenGL 扩展库
glXCreateContextAttribsARBProc glXCreateContextAttribsARB = (glXCreateContextAttribsARBProc)glXGetProcAddressARB((const GLubyte*)"glXCreateContextAttribsARB");
if (glXCreateContextAttribsARB == NULL) {
printf("Error: cannot load glXCreateContextAttribsARB\n");
return 1;
}
const char *glExtensions = glXQueryExtensionsString(display, screen);
if (strstr(glExtensions, "GLX_ARB_create_context") == NULL) {
printf("Error: GLX_ARB_create_context not supported\n");
return 1;
}
// 3. 为 OpenGL 上下文设置像素格式
typedef GLXContext (*glXCreateContextAttribsARBProc)(Display*, GLXFBConfig, GLXContext, Bool, const int*);
int contextAttributes[] = {
GLX_CONTEXT_MAJOR_VERSION_ARB, 3,
GLX_CONTEXT_MINOR_VERSION_ARB, 3,
None
};
GLXFBConfig fbConfig = 0;
int fbConfigsCount = 0;
GLXFBConfig *fbConfigs = glXChooseFBConfig(display, screen, visualAttributes, &fbConfigsCount);
if (fbConfigs == NULL || fbConfigsCount == 0) {
printf("Error: cannot find suitable framebuffer configuration\n");
return 1;
}
for (int i = 0; i < fbConfigsCount; i++) {
XVisualInfo *vi = glXGetVisualFromFBConfig(display, fbConfigs[i]);
if (vi != NULL && vi->depth == visual->depth) {
fbConfig = fbConfigs[i];
break;
}
}
if (fbConfig == 0) {
printf("Error: cannot find suitable framebuffer configuration\n");
return 1;
}
glContext = glXCreateContextAttribsARB(display, fbConfig, 0, True, contextAttributes);
if (glContext == NULL) {
printf("Error: cannot create OpenGL context\n");
return 1;
}
// 4. 激活 OpenGL 上下文
glXMakeCurrent(display, window, glContext);
// 进入主循环
while (1) {
XEvent event;
XNextEvent(display, &event);
switch (event.type) {
case Expose:
glClearColor(0, 0, 0, 1);
glClear(GL_COLOR_BUFFER_BIT);
glXSwapBuffers(display, window);
break;
case KeyPress:
XCloseDisplay(display);
return 0;
}
}
}
```
以上代码使用了 X11 和 GLX 库来创建窗口和 OpenGL 上下文,并在主循环中使用 OpenGL 渲染窗口。注意代码中的注释和错误处理。代码中还使用了 GLX_ARB_create_context 扩展来创建 OpenGL 3.3 上下文,如果需要使用其他版本的 OpenGL,可以修改相应的参数。
linux x11桌面环境
Linux X11桌面环境是一种以位图方式显示的软件窗口系统环境。它提供了一个图形用户界面,允许用户通过图形界面与操作系统进行交互。X窗口系统是一种非常灵活和可定制的系统,可以在各种Linux发行版中使用。
X11桌面环境包括多个具有不同特点的桌面环境,如GNOME、KDE、Xfce、LXDE等。每个桌面环境都有自己独特的外观和功能,以满足不同用户的需求和偏好。
在安装Linux发行版时,通常可以选择安装特定的桌面环境,或者可以在已安装的系统上安装其他桌面环境。安装完成后,用户可以在登录界面选择所需的桌面环境,以获得他们想要的视觉效果和功能。
相关推荐
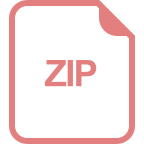
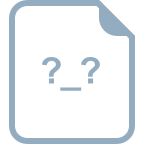












