let html = '<br\><br\>' + ' <div class="form-group" style="display: flex;">\n' + ' <label class="control-label " style="margin-right: 4px;">测试日志1</label>\n' + ' <div >\n' + ' <textarea style="height: 240px; width: 720px;" class="form-control"\n' + ' id="test1" name="test1" rows="5"></textarea>\n' + ' </div>\n' + ' </div>\n' + ' <br\> ' + ' <div class="form-group" style="display: flex;">\n' + ' <label class="control-label " style="margin-right: 4px;">测试日志2</label>\n' + ' <div >\n' + ' <textarea style="height: 240px; width: 720px;" class="form-control"\n' + ' id="test2" name="test2" rows="5"></textarea>\n' + ' </div>\n' + '</div>'; 表单校验是否为空
时间: 2023-10-23 18:11:49 浏览: 108
你可以使用 JavaScript 来进行表单校验,以下是一个示例代码:
```javascript
// 获取表单中的两个文本框
const test1Input = document.getElementById('test1');
const test2Input = document.getElementById('test2');
// 判断两个文本框是否为空
if (test1Input.value.trim() === '') {
alert('测试日志1不能为空!');
return false;
}
if (test2Input.value.trim() === '') {
alert('测试日志2不能为空!');
return false;
}
// 如果都不为空,则提交表单
document.forms[0].submit();
```
这段代码首先获取了表单中两个文本框的元素,然后使用 `trim()` 方法去除两端的空格,判断是否为空。如果为空,则弹出提示框并返回 `false`,否则提交表单。你需要将这段代码放在表单提交的事件处理函数中。
相关问题
vue+element 柱状图
好的,下面是基于 Vue.js 和 Element UI 组件库实现的柱状图示例:
1. 安装 Element UI 组件库并按需引入相应组件:
```
npm i element-ui -S
```
```js
// 在 main.js 中按需引入组件
import Vue from 'vue';
import {
Button,
Select,
Option,
Row,
Col,
Input,
Form,
FormItem,
Loading,
Message,
MessageBox,
Notification,
Container,
Header,
Aside,
Main,
Menu,
Submenu,
MenuItem,
MenuItemGroup,
Breadcrumb,
BreadcrumbItem,
Card,
Table,
TableColumn,
Pagination,
Dialog,
Dropdown,
DropdownMenu,
DropdownItem,
Tabs,
TabPane,
Checkbox,
CheckboxGroup,
Radio,
RadioGroup,
Switch,
DatePicker,
TimePicker,
Upload,
Steps,
Step,
Carousel,
CarouselItem,
Tooltip,
Popover,
Alert,
Tag,
Badge,
Progress,
Tree,
Cascader,
Avatar,
Divider,
Image,
Popconfirm,
Slider,
Transfer,
ColorPicker,
Scrollbar,
Collapse,
CollapseItem
} from 'element-ui';
Vue.use(Button);
Vue.use(Select);
Vue.use(Option);
Vue.use(Row);
Vue.use(Col);
Vue.use(Input);
Vue.use(Form);
Vue.use(FormItem);
Vue.use(Loading.directive);
Vue.prototype.$loading = Loading.service;
Vue.prototype.$message = Message;
Vue.prototype.$msgbox = MessageBox;
Vue.prototype.$alert = MessageBox.alert;
Vue.prototype.$confirm = MessageBox.confirm;
Vue.prototype.$prompt = MessageBox.prompt;
Vue.prototype.$notify = Notification;
Vue.use(Container);
Vue.use(Header);
Vue.use(Aside);
Vue.use(Main);
Vue.use(Menu);
Vue.use(Submenu);
Vue.use(MenuItem);
Vue.use(MenuItemGroup);
Vue.use(Breadcrumb);
Vue.use(BreadcrumbItem);
Vue.use(Card);
Vue.use(Table);
Vue.use(TableColumn);
Vue.use(Pagination);
Vue.use(Dialog);
Vue.use(Dropdown);
Vue.use(DropdownMenu);
Vue.use(DropdownItem);
Vue.use(Tabs);
Vue.use(TabPane);
Vue.use(Checkbox);
Vue.use(CheckboxGroup);
Vue.use(Radio);
Vue.use(RadioGroup);
Vue.use(Switch);
Vue.use(DatePicker);
Vue.use(TimePicker);
Vue.use(Upload);
Vue.use(Steps);
Vue.use(Step);
Vue.use(Carousel);
Vue.use(CarouselItem);
Vue.use(Tooltip);
Vue.use(Popover);
Vue.use(Alert);
Vue.use(Tag);
Vue.use(Badge);
Vue.use(Progress);
Vue.use(Tree);
Vue.use(Cascader);
Vue.use(Avatar);
Vue.use(Divider);
Vue.use(Image);
Vue.use(Popconfirm);
Vue.use(Slider);
Vue.use(Transfer);
Vue.use(ColorPicker);
Vue.use(Scrollbar);
Vue.use(Collapse);
Vue.use(CollapseItem);
```
2. 在组件中使用 echarts 绘制柱状图并绑定数据:
```html
<template>
<div class="chart-container">
<div ref="chart" class="chart"></div>
</div>
</template>
<script>
import echarts from 'echarts';
export default {
name: 'BarChart',
data() {
return {
chartData: [
{ month: '一月', sales: 1000 },
{ month: '二月', sales: 1200 },
{ month: '三月', sales: 800 },
{ month: '四月', sales: 1500 },
{ month: '五月', sales: 1300 },
{ month: '六月', sales: 1700 },
{ month: '七月', sales: 900 }
]
}
},
mounted() {
// 基于准备好的dom,初始化echarts实例
let myChart = echarts.init(this.$refs.chart);
// 指定图表的配置项和数据
let option = {
title: {
text: '月度销售额',
subtext: '单位:元'
},
tooltip: {},
xAxis: {
data: this.chartData.map(item => item.month),
axisLabel: {
interval: 0, // 强制显示所有刻度标签
rotate: 45, // 旋转角度
margin: 10 // 刻度标签与轴线之间的距离
}
},
yAxis: {},
series: [{
name: '销售额',
type: 'bar',
data: this.chartData.map(item => item.sales),
itemStyle: {
// 柱形图圆角
barBorderRadius: 5
}
}]
};
// 使用刚指定的配置项和数据显示图表。
myChart.setOption(option);
}
}
</script>
<style scoped>
.chart-container {
width: 100%;
height: 400px;
display: flex;
justify-content: center;
align-items: center;
}
.chart {
width: 80%;
height: 80%;
}
</style>
```
以上就是基于 Vue.js 和 Element UI 组件库实现的柱状图示例。其中,echarts 是一个非常强大的数据可视化库,可以通过配置项和数据来绘制各种类型的图表。
编写一个Angular程序,使用ng-zorro-antd组件库中的7种组件实现相应的功能。
好的!我可以为您提供一个简单的示例程序,它使用了ng-zorro-antd组件库中的七种组件:
1. Button(按钮)
2. Input(输入框)
3. Checkbox(复选框)
4. Radio(单选框)
5. Select(下拉选择框)
6. Datepicker(日期选择器)
7. Table(表格)
以下是示例程序的代码:
app.module.ts
```typescript
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { NzButtonModule, NzInputModule, NzCheckboxModule, NzRadioModule, NzSelectModule, NzDatePickerModule, NzTableModule } from 'ng-zorro-antd';
import { AppComponent } from './app.component';
@NgModule({
imports: [ BrowserModule, FormsModule, BrowserAnimationsModule, NzButtonModule, NzInputModule, NzCheckboxModule, NzRadioModule, NzSelectModule, NzDatePickerModule, NzTableModule ],
declarations: [ AppComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
```
app.component.ts
```typescript
import { Component } from '@angular/core';
interface User {
name: string;
age: number;
gender: string;
}
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
users: User[] = [
{ name: '张三', age: 20, gender: '男' },
{ name: '李四', age: 25, gender: '女' },
{ name: '王五', age: 30, gender: '男' },
];
selectedUser: User = null;
newUser: User = { name: '', age: null, gender: '' };
genderOptions = [ { label: '男', value: '男' }, { label: '女', value: '女' } ];
selectUser(user: User): void {
this.selectedUser = user;
}
addUser(): void {
this.users.push(this.newUser);
this.newUser = { name: '', age: null, gender: '' };
}
deleteUser(user: User): void {
const index = this.users.indexOf(user);
if (index >= 0) {
this.users.splice(index, 1);
this.selectedUser = null;
}
}
}
```
app.component.html
```html
<h1>用户管理</h1>
<div style="display: flex; flex-direction: row;">
<div style="flex: 1;">
<nz-table [nzData]="users" [nzFrontPagination]="false" [nzBordered]="true" [nzSize]="'middle'" (nzClick)="selectUser($event)">
<thead>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let user of users">
<td>{{ user.name }}</td>
<td>{{ user.age }}</td>
<td>{{ user.gender }}</td>
</tr>
</tbody>
</nz-table>
<div style="margin-top: 16px;">
<button nz-button [nzType]="'primary'" [disabled]="selectedUser === null" (click)="deleteUser(selectedUser)">删除</button>
</div>
</div>
<div style="flex: 1; margin-left: 16px;">
<form #userForm="ngForm">
<div>
<label>姓名:</label>
<input nz-input [(ngModel)]="newUser.name" name="name" required>
</div>
<div>
<label>年龄:</label>
<input nz-input [(ngModel)]="newUser.age" name="age" type="number" required>
</div>
<div>
<label>性别:</label>
<nz-radio-group [(ngModel)]="newUser.gender" name="gender" required>
<label nz-radio *ngFor="let option of genderOptions" [nzValue]="option.value">{{ option.label }}</label>
</nz-radio-group>
</div>
<div style="margin-top: 16px;">
<button nz-button [nzType]="'primary'" [disabled]="!userForm.valid" (click)="addUser()">添加</button>
</div>
</form>
</div>
</div>
```
这个示例程序演示了如何使用ng-zorro-antd组件库中的七种组件来实现一个简单的用户管理功能,包括显示用户列表、选择用户、添加用户和删除用户。您可以参考这个示例程序来编写自己的Angular程序,使用ng-zorro-antd组件库中的各种组件来实现您想要的功能。
阅读全文
相关推荐
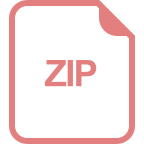
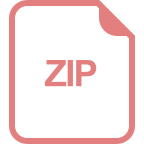
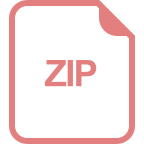
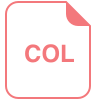
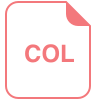
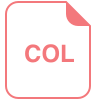
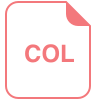
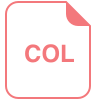

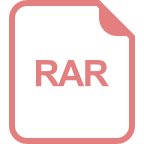
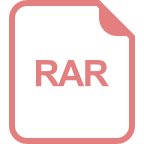
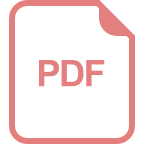
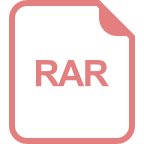