基于Pytorch实现多层感知机多输入的回归预测,数据从本地导入,并详细解释各参数含义及如何调参,数据集一共有15000个数据点、13个特征
时间: 2024-04-08 12:35:01 浏览: 176
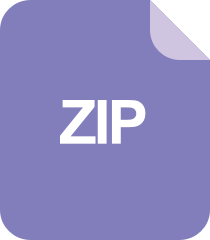
MLP识别MNIST手写数字数据集(Pytorch)
对于基于PyTorch的多层感知机(Multilayer Perceptron,MLP)多输入的回归预测问题,可以按照以下步骤进行实现:
1. 导入必要的库和模块:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
```
2. 加载和预处理数据:
```python
# 读取数据
data = pd.read_csv('data.csv')
# 分离特征和标签
X = data.iloc[:, :13].values
y = data.iloc[:, 13].values
# 数据标准化
scaler = StandardScaler()
X = scaler.fit_transform(X)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
3. 定义多层感知机模型:
```python
class MLP(nn.Module):
def __init__(self, input_size):
super(MLP, self).__init__()
self.fc1 = nn.Linear(input_size, 64)
self.fc2 = nn.Linear(64, 32)
self.fc3 = nn.Linear(32, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.relu(self.fc2(x))
x = self.fc3(x)
return x
model = MLP(input_size=13)
```
4. 定义损失函数和优化器:
```python
criterion = nn.MSELoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
```
5. 定义训练循环:
```python
num_epochs = 100
for epoch in range(num_epochs):
model.train()
inputs = torch.tensor(X_train, dtype=torch.float32)
targets = torch.tensor(y_train, dtype=torch.float32)
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, targets)
loss.backward()
optimizer.step()
if (epoch+1) % 10 == 0:
print(f'Epoch {epoch+1}/{num_epochs}, Loss: {loss.item():.4f}')
```
在训练循环中,我们将输入和目标转换为PyTorch张量,并执行前向传播、计算损失、反向传播和优化器的更新。
6. 模型评估:
```python
model.eval()
with torch.no_grad():
inputs = torch.tensor(X_test, dtype=torch.float32)
targets = torch.tensor(y_test, dtype=torch.float32)
outputs = model(inputs)
test_loss = criterion(outputs, targets)
print(f'Test Loss: {test_loss.item():.4f}')
```
这段代码计算了测试集上的损失,用于评估模型的性能。
参数含义和调参解释如下:
- `input_size`:输入特征的维度,本例中为13。
- `nn.Linear(input_size, output_size)`:定义一个线性层,其中`input_size`为输入特征的维度,`output_size`为输出特征的维度。
- `nn.ReLU()`:ReLU激活函数,用于引入非线性变换。
- `nn.MSELoss()`:均方误差损失函数,用于回归问题。
- `optim.Adam(model.parameters(), lr=0.001)`:Adam优化器,用于参数更新。
- `num_epochs`:训练的轮数,可以根据实际情况进行调整。
- `test_size=0.2`:将数据集划分为训练集和测试集,测试集占总数据集的20%。
- `optimizer.zero_grad()`:梯度清零,防止梯度累积。
- `loss.backward()`:计算损失相对于模型参数的梯度。
- `optimizer.step()`:更新模型参数。
调参时可以尝试调整以下参数:
- 学习率(lr):控制参数更新的步长,如果学习率过大,模型可能无法收敛;如果学习率过小,模型收敛速度可能过慢。
- 隐藏层的神经元数量:可以尝试增加或减少隐藏层的神经元数量,以寻找合适的模型复杂度。
- 训练轮数(num_epochs):增加训练轮数可能会提高模型性能,但也容易导致过拟合。
请注意,以上代码仅为示例,实际调参时还需根据具体问题和数据集的特点进行调整。
阅读全文
相关推荐
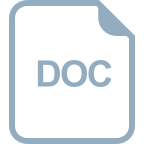
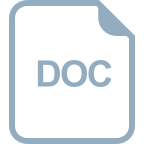

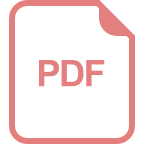
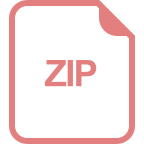
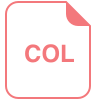
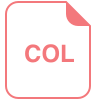
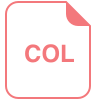
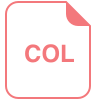
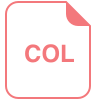
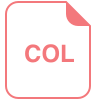
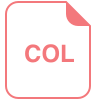
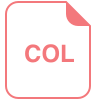
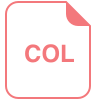
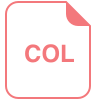
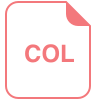
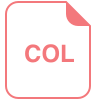
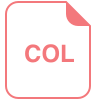