dpos算法的代码实现
时间: 2023-05-29 08:03:28 浏览: 179
由于dpos算法是一种共识算法,需要在区块链的整个系统中进行实现,因此代码实现比较复杂,需要考虑多个方面的因素,包括选举、投票、验证等。
以下是dpos算法的大致代码实现:
1. 定义节点
节点包括地址、公钥、私钥等信息,同时还需要定义节点的角色,包括候选节点和验证节点。
```python
class Node:
def __init__(self, address, public_key, private_key, role):
self.address = address
self.public_key = public_key
self.private_key = private_key
self.role = role
def __str__(self):
return '{}: {} ({})'.format(self.role, self.address, self.public_key)
```
2. 定义候选节点和验证节点
候选节点是在区块链中申请成为验证节点的节点,如果被选中则成为验证节点,否则继续竞选。验证节点是负责验证交易和生成新区块的节点。
```python
class Candidate(Node):
def __init__(self, address, public_key, private_key):
super().__init__(address, public_key, private_key, 'Candidate')
self.votes = 0
class Validator(Node):
def __init__(self, address, public_key, private_key):
super().__init__(address, public_key, private_key, 'Validator')
```
3. 定义投票
投票是指候选节点和其他验证节点对候选节点的支持,投票需要考虑到节点的权重和投票时间等因素。
```python
class Vote:
def __init__(self, voter, candidate, weight, timestamp):
self.voter = voter
self.candidate = candidate
self.weight = weight
self.timestamp = timestamp
```
4. 定义选举
选举是指在每个区块的生成过程中,根据候选节点的得票数选出一定数量的验证节点。选举需要根据节点的权重和得票数进行排序,然后选出前面的节点作为验证节点。
```python
class Election:
def __init__(self, candidates, validators):
self.candidates = candidates
self.validators = validators
def run_election(self):
sorted_candidates = sorted(self.candidates, key=lambda x: x.votes, reverse=True)
num_validators = len(self.validators)
for i in range(min(num_validators, len(sorted_candidates))):
self.validators[i] = Validator(sorted_candidates[i].address, sorted_candidates[i].public_key, sorted_candidates[i].private_key)
```
5. 定义验证
验证是指验证交易是否合法和生成新区块。在dpos算法中,只有验证节点有权生成新区块,而其他节点只能提交交易和投票。
```python
class Block:
def __init__(self, transactions, previous_hash):
self.transactions = transactions
self.previous_hash = previous_hash
self.timestamp = time.time()
self.validator = None
self.signature = None
def sign(self, private_key):
self.signature = private_key.sign(str(self).encode(), padding.PSS(mgf=padding.MGF1(hashes.SHA256()), salt_length=padding.PSS.MAX_LENGTH), hashes.SHA256())
def validate(self):
public_key = self.validator.public_key
signature = self.signature
message = str(self).encode()
public_key.verify(signature, message, padding.PSS(mgf=padding.MGF1(hashes.SHA256()), salt_length=padding.PSS.MAX_LENGTH), hashes.SHA256())
class ValidatorNode(Node):
def __init__(self, address, public_key, private_key):
super().__init__(address, public_key, private_key, 'Validator')
def validate_transaction(self, transaction):
# 验证交易是否合法
pass
def validate_block(self, block):
# 验证区块是否合法
pass
def generate_block(self, transactions, previous_hash):
# 生成新区块
block = Block(transactions, previous_hash)
block.validator = self
block.sign(self.private_key)
return block
```
6. 实现整个算法
最后,将上述代码组合起来实现整个dpos算法。
```python
class DPOS:
def __init__(self, validators, candidates):
self.validators = validators
self.candidates = candidates
self.election = Election(self.candidates, self.validators)
def vote(self, voter, candidate, weight):
# 投票
pass
def submit_transaction(self, transaction):
# 提交交易
pass
def generate_block(self, transactions):
# 生成新区块
pass
def run(self):
# 运行dpos算法
pass
```
以上是dpos算法的大致代码实现,实际实现过程中还需要考虑到更多的细节和安全性问题。
阅读全文
相关推荐
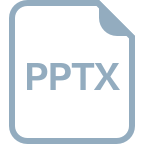
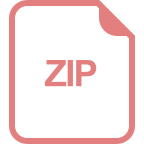
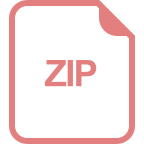
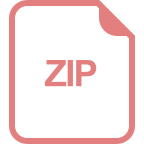
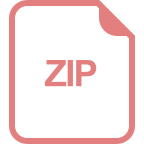
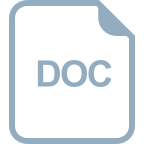
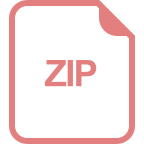
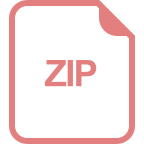
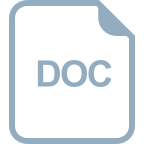
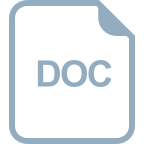
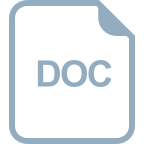
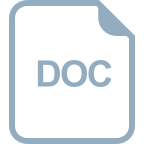
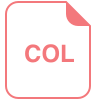
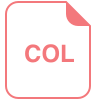
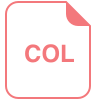
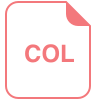