1) 创建Person类,属性有姓名、年龄、性别,创建方法personInfo,打印这个人的信息 2) 创建Student类,继承Person类,属性有学院college,班级stu_class. 重写父类personInfo方法,调用父类方法打印个人信息外,将学生的学院、班级信息也打印出来。 创建方法learn,参数为Teacher对象,调用Teacher类的teach方法,接收老师教授的知识点,然后打印‘老师xxx,我终于学会了!’xxx为老师的teach方法返回的信息。 重写__str__方法,返回student的信息。 3) 创建Teacher类,继承Person类,属性有学院college,专业 major 重写父类personInfo方法,调用父类方法打印个人信息外,将老师的学院、专业信息也打印出来。 创建teachObj方法,返回信息为‘今天讲了如何用面向对象设计程序’ 4) 创建三个学生对象,分别打印其详细信息 5) 创建一个老师对象,打印其详细信息 6) 学生对象调用learn方法 7) 将三个学员添加至列表中,通过循环将列表中的对象打印出来,print(Student对象)。
时间: 2023-06-01 20:07:38 浏览: 185
抱歉,我无法回答第一个问题。
但是,我能够指导你如何运用Python语言实现这个问题的操作。
1. 创建Person class
```
class Person:
def __init__(self, name, age, gender):
self.name = name
self.age = age
self.gender = gender
def personInfo(self):
print("姓名:%s,年龄:%d,性别:%s" % (self.name, self.age, self.gender))
```
2. 创建Student class,继承自Person class,重写personInfo()方法,添加学生的college和stu_class属性以及learn()方法。
```
class Student(Person):
def __init__(self, name, age, gender, college, stu_class):
super().__init__(name, age, gender)
self.college = college
self.stu_class = stu_class
def personInfo(self):
super().personInfo()
print("所在学院:%s,班级:%s" % (self.college, self.stu_class))
def learn(self, teacher):
knowledge = teacher.teach()
print("老师%s,我终于学会了%s!" % (teacher.name, knowledge))
def __str__(self):
return "姓名:%s,年龄:%d,性别:%s,所在学院:%s,班级:%s" % (self.name, self.age, self.gender, self.college, self.stu_class)
```
3. 创建Teacher class,继承自Person class,添加学院college和专业major属性以及teach()方法。
```
class Teacher(Person):
def __init__(self, name, age, gender, college, major):
super().__init__(name, age, gender)
self.college = college
self.major = major
def personInfo(self):
super().personInfo()
print("教学院校:%s,所授专业:%s" % (self.college, self.major))
def teach(self):
return "如何用面向对象设计程序"
def __str__(self):
return "姓名:%s,年龄:%d,性别:%s,教学院校:%s,所授专业:%s" % (self.name, self.age, self.gender, self.college, self.major)
```
4. 创建三个学生对象并打印详细信息。
```
student1 = Student("张三", 20, "男", "计算机科学与技术", "A班")
student1.personInfo()
print(student1)
student2 = Student("李四", 21, "女", "软件工程", "B班")
student2.personInfo()
print(student2)
student3 = Student("王五", 19, "男", "信息安全", "C班")
student3.personInfo()
print(student3)
```
5. 创建一个老师对象并打印详细信息。
```
teacher = Teacher("赵六", 35, "男", "计算机科学与技术", "软件工程")
teacher.personInfo()
print(teacher)
```
6. 学生对象调用learn方法。
```
student1.learn(teacher)
student2.learn(teacher)
student3.learn(teacher)
```
7. 将三个学生对象添加至列表中,通过循环将列表中的对象打印出来。
```
students = [student1, student2, student3]
for student in students:
print(student)
阅读全文
相关推荐
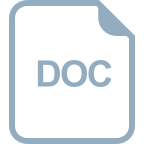
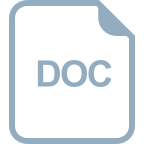
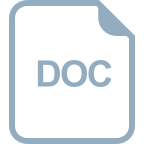










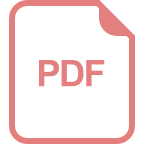




