括号匹配问题 栈c语言
时间: 2023-05-20 13:05:02 浏览: 122
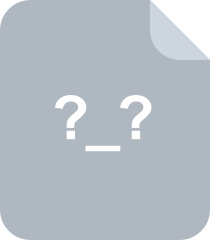
C语言版数据结构栈中括号匹配问题

好的,让我来回答你的问题。括号匹配问题是一个经典的栈应用问题,它可以用栈来解决。在C语言中,可以使用数组来模拟栈的操作,具体实现可以参考以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
int top = -1;
char stack[MAX_SIZE];
void push(char c) {
if (top == MAX_SIZE - 1) {
printf("Stack overflow!\n");
exit(1);
}
stack[++top] = c;
}
char pop() {
if (top == -1) {
printf("Stack underflow!\n");
exit(1);
}
return stack[top--];
}
int is_matching_pair(char left, char right) {
if (left == '(' && right == ')')
return 1;
else if (left == '{' && right == '}')
return 1;
else if (left == '[' && right == ']')
return 1;
else
return 0;
}
int is_balanced(char *exp) {
int i;
for (i = 0; i < strlen(exp); i++) {
if (exp[i] == '(' || exp[i] == '{' || exp[i] == '[')
push(exp[i]);
else if (exp[i] == ')' || exp[i] == '}' || exp[i] == ']') {
if (top == -1 || !is_matching_pair(pop(), exp[i]))
return 0;
}
}
return (top == -1);
}
int main() {
char exp[MAX_SIZE];
printf("Enter an expression: ");
scanf("%s", exp);
if (is_balanced(exp))
printf("The expression is balanced!\n");
else
printf("The expression is not balanced!\n");
return 0;
}
```
这段代码实现了一个简单的括号匹配检查程序,它可以检查输入的表达式中的括号是否匹配。如果匹配,程序输出"The expression is balanced!",否则输出"The expression is not balanced!"。
希望这个回答能够帮到你!
阅读全文
相关推荐
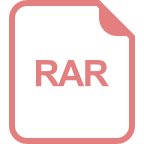


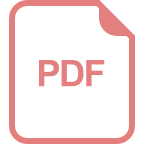
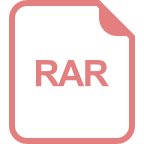
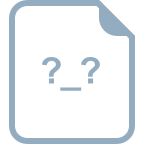






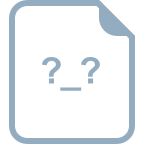
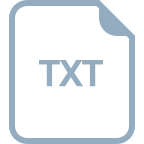