c语言对结构体的读取与写入
时间: 2024-05-01 11:22:42 浏览: 21
在C语言中,结构体是一种用户自定义的数据类型,它可以包含不同类型的数据成员。对于结构体的读取和写入,可以使用结构体变量及其成员进行操作。
下面是一些示例代码:
1. 结构体的定义
```c
struct student {
char name[20];
int age;
float score;
};
```
2. 结构体的读取
```c
struct student stu;
printf("请输入学生姓名:");
scanf("%s", stu.name);
printf("请输入学生年龄:");
scanf("%d", &stu.age);
printf("请输入学生成绩:");
scanf("%f", &stu.score);
printf("学生姓名:%s\n", stu.name);
printf("学生年龄:%d\n", stu.age);
printf("学生成绩:%f\n", stu.score);
```
3. 结构体的写入
```c
struct student stu = {"Tom", 18, 90.5};
printf("学生姓名:%s\n", stu.name);
printf("学生年龄:%d\n", stu.age);
printf("学生成绩:%f\n", stu.score);
```
4. 结构体数组的读取和写入
```c
struct student stu[3];
for(int i = 0; i < 3; i++) {
printf("请输入第%d个学生的姓名:", i+1);
scanf("%s", stu[i].name);
printf("请输入第%d个学生的年龄:", i+1);
scanf("%d", &stu[i].age);
printf("请输入第%d个学生的成绩:", i+1);
scanf("%f", &stu[i].score);
}
for(int i = 0; i < 3; i++) {
printf("第%d个学生的姓名:%s\n", i+1, stu[i].name);
printf("第%d个学生的年龄:%d\n", i+1, stu[i].age);
printf("第%d个学生的成绩:%f\n", i+1, stu[i].score);
}
```
希望这些示例代码可以帮助你理解如何在C语言中读取和写入结构体。
相关推荐
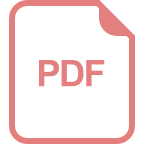
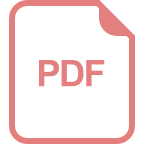
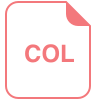
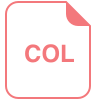
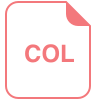
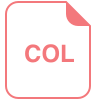
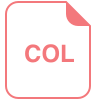









