c#repository用法
时间: 2023-08-26 19:05:20 浏览: 43
C# 中的 Repository 是一种设计模式,用于将数据存取代码从业务逻辑中分离出来。它通过提供一组通用的接口和方法,来实现对数据的 CRUD(创建、读取、更新、删除)操作。
以下是一个简单的 C# Repository 的示例:
```csharp
public interface IRepository<T> where T : class
{
IEnumerable<T> GetAll();
T GetById(int id);
void Add(T entity);
void Update(T entity);
void Delete(T entity);
}
public class Repository<T> : IRepository<T> where T : class
{
private readonly DbContext _context;
private readonly DbSet<T> _entities;
public Repository(DbContext context)
{
_context = context;
_entities = context.Set<T>();
}
public IEnumerable<T> GetAll()
{
return _entities.ToList();
}
public T GetById(int id)
{
return _entities.Find(id);
}
public void Add(T entity)
{
_entities.Add(entity);
_context.SaveChanges();
}
public void Update(T entity)
{
_entities.Update(entity);
_context.SaveChanges();
}
public void Delete(T entity)
{
_entities.Remove(entity);
_context.SaveChanges();
}
}
```
这个示例中,我们定义了一个 IRepository 接口和一个 Repository 类,Repository 类实现了 IRepository 接口的方法。在构造函数中,我们创建了一个 DbContext 对象并初始化了一个 DbSet,这是 Entity Framework 中的一种数据访问方式。
在 GetAll 方法中,我们使用了 _entities.ToList() 来获取 T 类型的所有实体对象。在 GetById 方法中,我们使用了 _entities.Find(id) 来获取指定 id 的实体对象。在 Add、Update 和 Delete 方法中,我们使用了 _entities.Add(entity)、_entities.Update(entity) 和 _entities.Remove(entity) 来添加、更新和删除实体对象,并使用 _context.SaveChanges() 来保存更改。
使用 Repository 的好处之一是,我们可以轻松地为不同的实体类型创建不同的 Repository 实现,并使用它们来访问和操作数据。因此,Repository 是一个非常有用的数据访问模式。
相关推荐
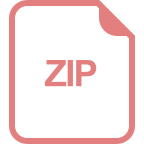
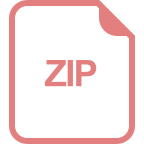
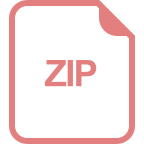













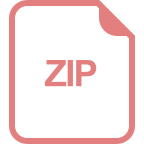
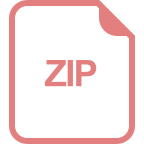