用Microsoft Access作为DBMS,建立数据库和数据表来实现对一个简易的英汉电子词典进行存储和管理,这个简易电子词典中的内容至少包括:英文单词名、词性、汉语释义、例句(其它信息可自行扩充)。请编写图形界面的Java Application,完成对电子词典中英文单词的查找、新词的添加、单词的修改、删除等功能。并提供一个main方法实现
时间: 2024-03-23 17:35:56 浏览: 32
好的,我可以帮您解答这个问题。
首先,您需要在Microsoft Access中创建一个数据库和数据表,包括英文单词名、词性、汉语释义、例句等字段。然后,您可以使用Java中的JDBC API来连接和操作这个数据库。
以下是一个示例程序,其中包含了电子词典的查找、添加、修改和删除功能:
```java
import java.sql.*;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class DictionaryApp extends JFrame implements ActionListener {
private static final String DB_URL = "jdbc:ucanaccess://dictionary.accdb";
private static final String DB_USER = "";
private static final String DB_PASSWORD = "";
private static final String[] PARTS_OF_SPEECH = {"n.", "v.", "adj.", "adv."};
private JTextField wordField, meaningField, exampleField;
private JComboBox<String> partOfSpeechBox;
private JButton addButton, updateButton, deleteButton, searchButton;
private Connection conn;
private Statement stmt;
private ResultSet rs;
public DictionaryApp() {
super("Dictionary");
// Create GUI components
JLabel wordLabel = new JLabel("Word:");
wordField = new JTextField(20);
JLabel partOfSpeechLabel = new JLabel("Part of speech:");
partOfSpeechBox = new JComboBox<>(PARTS_OF_SPEECH);
JLabel meaningLabel = new JLabel("Meaning:");
meaningField = new JTextField(20);
JLabel exampleLabel = new JLabel("Example:");
exampleField = new JTextField(20);
addButton = new JButton("Add");
addButton.addActionListener(this);
updateButton = new JButton("Update");
updateButton.addActionListener(this);
deleteButton = new JButton("Delete");
deleteButton.addActionListener(this);
searchButton = new JButton("Search");
searchButton.addActionListener(this);
// Add components to content pane
Container contentPane = getContentPane();
contentPane.setLayout(new GridLayout(5, 2));
contentPane.add(wordLabel);
contentPane.add(wordField);
contentPane.add(partOfSpeechLabel);
contentPane.add(partOfSpeechBox);
contentPane.add(meaningLabel);
contentPane.add(meaningField);
contentPane.add(exampleLabel);
contentPane.add(exampleField);
contentPane.add(addButton);
contentPane.add(updateButton);
contentPane.add(deleteButton);
contentPane.add(searchButton);
// Connect to database
try {
conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
stmt = conn.createStatement();
} catch (SQLException e) {
e.printStackTrace();
System.exit(1);
}
// Set window properties
setSize(400, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public static void main(String[] args) {
new DictionaryApp();
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
addWord();
} else if (e.getSource() == updateButton) {
updateWord();
} else if (e.getSource() == deleteButton) {
deleteWord();
} else if (e.getSource() == searchButton) {
searchWord();
}
}
private void addWord() {
String word = wordField.getText();
String partOfSpeech = (String) partOfSpeechBox.getSelectedItem();
String meaning = meaningField.getText();
String example = exampleField.getText();
if (!word.isEmpty() && !meaning.isEmpty()) {
try {
String sql = "INSERT INTO dictionary (word, part_of_speech, meaning, example) " +
"VALUES ('" + word + "', '" + partOfSpeech + "', '" + meaning + "', '" + example + "')";
stmt.executeUpdate(sql);
JOptionPane.showMessageDialog(this, "Word added successfully.");
clearFields();
} catch (SQLException e) {
e.printStackTrace();
JOptionPane.showMessageDialog(this, "Error adding word: " + e.getMessage());
}
} else {
JOptionPane.showMessageDialog(this, "Please enter word and meaning.");
}
}
private void updateWord() {
String word = wordField.getText();
String partOfSpeech = (String) partOfSpeechBox.getSelectedItem();
String meaning = meaningField.getText();
String example = exampleField.getText();
if (!word.isEmpty() && !meaning.isEmpty()) {
try {
String sql = "UPDATE dictionary SET part_of_speech='" + partOfSpeech + "', meaning='" + meaning + "', " +
"example='" + example + "' WHERE word='" + word + "'";
int rowsUpdated = stmt.executeUpdate(sql);
if (rowsUpdated > 0) {
JOptionPane.showMessageDialog(this, "Word updated successfully.");
clearFields();
} else {
JOptionPane.showMessageDialog(this, "Word not found.");
}
} catch (SQLException e) {
e.printStackTrace();
JOptionPane.showMessageDialog(this, "Error updating word: " + e.getMessage());
}
} else {
JOptionPane.showMessageDialog(this, "Please enter word and meaning.");
}
}
private void deleteWord() {
String word = wordField.getText();
if (!word.isEmpty()) {
try {
String sql = "DELETE FROM dictionary WHERE word='" + word + "'";
int rowsDeleted = stmt.executeUpdate(sql);
if (rowsDeleted > 0) {
JOptionPane.showMessageDialog(this, "Word deleted successfully.");
clearFields();
} else {
JOptionPane.showMessageDialog(this, "Word not found.");
}
} catch (SQLException e) {
e.printStackTrace();
JOptionPane.showMessageDialog(this, "Error deleting word: " + e.getMessage());
}
} else {
JOptionPane.showMessageDialog(this, "Please enter word.");
}
}
private void searchWord() {
String word = wordField.getText();
if (!word.isEmpty()) {
try {
String sql = "SELECT part_of_speech, meaning, example FROM dictionary WHERE word='" + word + "'";
rs = stmt.executeQuery(sql);
if (rs.next()) {
String partOfSpeech = rs.getString("part_of_speech");
String meaning = rs.getString("meaning");
String example = rs.getString("example");
partOfSpeechBox.setSelectedItem(partOfSpeech);
meaningField.setText(meaning);
exampleField.setText(example);
} else {
JOptionPane.showMessageDialog(this, "Word not found.");
}
} catch (SQLException e) {
e.printStackTrace();
JOptionPane.showMessageDialog(this, "Error searching word: " + e.getMessage());
}
} else {
JOptionPane.showMessageDialog(this, "Please enter word.");
}
}
private void clearFields() {
wordField.setText("");
meaningField.setText("");
exampleField.setText("");
}
}
```
请注意,这只是一个示例程序,可能需要根据您的具体情况进行修改和扩展。此外,为了保证程序的稳定性和安全性,您还需要添加适当的错误处理和数据验证逻辑。
相关推荐
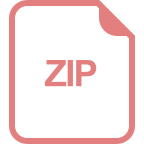
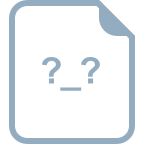




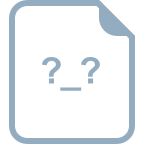
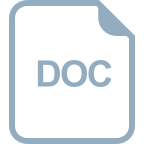
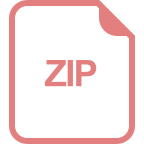
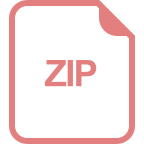
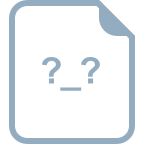
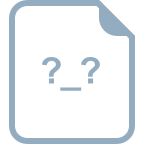
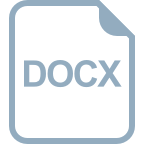
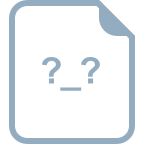
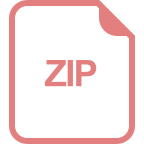