private static Date toDate(String str) { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); Date date = null; try { date = sdf.parse(str); } catch (ParseException e) { e.printStackTrace(); } return date; }解释说明一下该代码。
时间: 2023-07-15 08:13:45 浏览: 89
这段代码是一个静态方法,用于将字符串类型的日期转换为日期类型。具体说明如下:
1. 方法名称为 toDate,接收一个字符串类型的参数 str。
2. 创建一个 SimpleDateFormat 对象 sdf,指定日期格式为 "yyyy-MM-dd"。
3. 创建一个 Date 对象 date,初始值为 null。
4. 使用 try-catch 块,尝试将字符串类型的日期转换为 Date 类型的日期。如果转换成功,则将结果赋值给 date 对象;否则,打印异常信息。
5. 返回转换后的 Date 对象。
该方法通常用于将从前端传来的日期字符串转换为 Java 中的日期类型,以便进行日期比较、计算等操作。
相关问题
SimpleDateFormat sdf1 = new SimpleDateFormat("yyyy-MM-dd HH:mm");请详细解释每一部分
Sure!
`SimpleDateFormat` is a class in Java that is used to format and parse dates in a specific pattern.
`"yyyy-MM-dd HH:mm"` is the pattern used to format the date. Let's break it down:
- `yyyy` represents the year in four digits. For example, 2022.
- `-` is a separator character.
- `MM` represents the month in two digits. For example, 01 for January and 12 for December.
- `-` is another separator character.
- `dd` represents the day in two digits. For example, 01 for the 1st of the month and 31 for the last day of the month.
- ` ` (a space) is another separator character.
- `HH` represents the hour in 24-hour format. For example, 00 for midnight and 23 for 11 PM.
- `:` is a separator character.
- `mm` represents the minute in two digits. For example, 00 for the top of the hour and 59 for the last minute of the hour.
So `SimpleDateFormat sdf1 = new SimpleDateFormat("yyyy-MM-dd HH:mm");` creates a new `SimpleDateFormat` object called `sdf1` with the specified date pattern. This object can be used to format dates in this pattern or parse strings into dates using this pattern.
我有一段代码出错 SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); Date date1 = sdf.parse("2023-05-21 16:00:00"); Date date2 = sdf.parse("2023-05-22 16:00:00"); Criteria criteria = Criteria.where("monitorDate").in(date1, date2); Query query = Query.query(criteria).with(sort); query.addCriteria(criteria); 报错为g.springframework.data.mongodb.InvalidMongoDbApiUsageException: Due to limitations of the com.mongodb.BasicDocument, you can't add a second 'monitorDate' criteria. Query already contains '{ "monitorDate" : { "$in" : [{ "$date" : 1684656000000 }, { "$date" : 1684742400000 }] } }' at org.springframework.data.mongodb.core.query.Query.addCriteria(Query.java:103) 帮忙分析一下
这个错误是因为你在添加查询条件时重复添加了名为"monitorDate"的条件。在你的代码中,你已经通过`Criteria.where("monitorDate").in(date1, date2)`添加了一个名为"monitorDate"的查询条件,然后又通过`query.addCriteria(criteria)`再次添加了这个条件。
如果你只想在查询中添加一个名为"monitorDate"的条件,你可以这样做:
```
Criteria criteria = Criteria.where("monitorDate").in(date1, date2);
Query query = Query.query(criteria).with(sort);
```
如果你想添加多个条件,你可以创建一个`Criteria`对象,然后使用它来添加多个条件。例如:
```
Criteria criteria = new Criteria();
criteria.andOperator(
Criteria.where("monitorDate").in(date1, date2),
Criteria.where("someOtherField").is("someValue")
);
Query query = Query.query(criteria).with(sort);
```
这将在查询中添加名为"monitorDate"和"someOtherField"的两个条件。
阅读全文
相关推荐
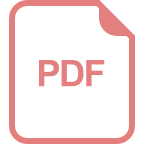
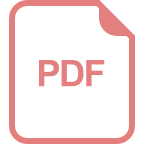













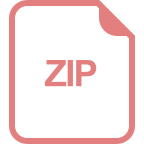
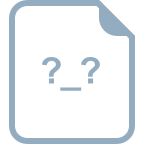