public void createTable(Individual individual){ CourseTable[] tables = new CourseTable[this.getNumTables()]; int[] chromsome = individual.getChromsome(); int chromesomePos = 0; int tablesIndex = 0; for (Task task : this.getTasksAsArray()) { tables[tablesIndex] = new CourseTable(); tables[tablesIndex].setTask(task); Timeslot timeslot = new Timeslot(); timeslot.setId(chromsome[chromesomePos]); tables[tablesIndex].setTimeslot(timeslot); chromesomePos++; Room room = new Room(); room.setId(chromsome[chromesomePos]); tables[tablesIndex].setRoom(room); chromesomePos++; tablesIndex++; } this.tables = tables; }
时间: 2023-07-14 13:12:52 浏览: 64
这段代码主要是用来创建一个课程表的,其中需要传入一个个体(Individual)作为参数,这个个体包含了一组染色体(chromosome),表示每个任务(Task)对应的时间槽(Timeslot)和教室(Room)。具体来说,代码中先创建了一个长度为getNumTables()的CourseTable数组,表示需要创建的课程表数量。然后通过循环遍历任务列表(this.getTasksAsArray()),为每个任务创建一个CourseTable对象,并设置对应的时间槽和教室。具体地,时间槽和教室的id分别从染色体数组中取出,所以需要一个变量chromesomePos来记录当前取到的染色体位置。最后将创建好的CourseTable数组赋值给对象的tables属性。
相关问题
public class Individual { private int[] chromsome; private double fitness = -1; public Individual(int[] chromsome) { this.chromsome = chromsome; } public Individual(Bootstrap bootstrap){ int numTables = bootstrap.getNumTables(); int chromosomeLength = numTables*2; int[] newChromosome = new int[chromosomeLength]; int chromosomeIndex = 0; for (Task task : bootstrap.getTasksAsArray()){ int timeslotId = bootstrap.getRandomTimeslot().getId(); newChromosome[chromosomeIndex] = timeslotId; chromosomeIndex++; int roomId = bootstrap.getRandomRoom().getId(); newChromosome[chromosomeIndex] = roomId; chromosomeIndex++; } this.chromsome = newChromosome; } public int[] getChromsome() { return chromsome; } public int getChromsomeLength() { return this.chromsome.length; } public double getFitness() { return fitness; } public void setFitness(double fitness) { this.fitness = fitness; } public int getGene(int offset) { return this.chromsome[offset]; } public void setGene(int offset, int gene) { this.chromsome[offset] = gene; } @Override public String toString() { String output = ""; for (int gene = 0; gene < this.chromsome.length; gene++) { output += this.chromsome[gene]; } return output; } }
这是一个基因个体类 Individual,它包含一个整型数组 chromsome ,表示一个个体的染色体,以及一个表示适应度的双精度浮点数 fitness。该类有两个构造函数,其中一个是从给定的 Bootstrap 对象生成一个个体,该对象包含了任务和时间段等信息。该构造函数将随机地为任务分配时间段和房间,并将它们存储在个体的染色体数组中。该类还提供了一些用于访问和修改个体染色体和适应度的方法,例如 getChromsome、setFitness、getGene、setGene 等。最后,该类还覆盖了 toString 方法,以打印出染色体的字符串表示形式。
阅读全文
相关推荐
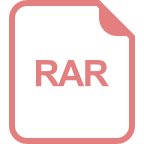
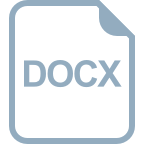
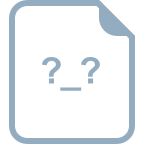
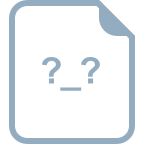
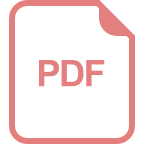
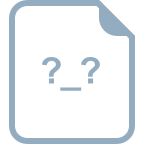
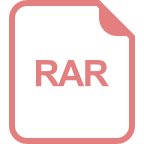
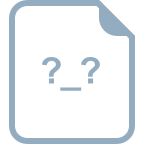
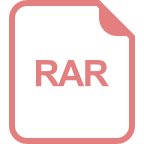
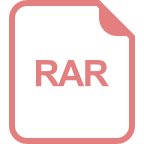
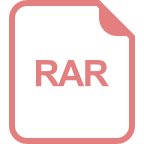
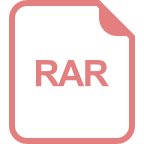
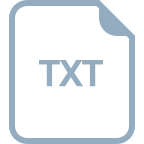
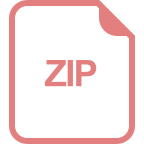
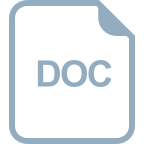
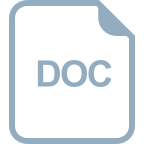
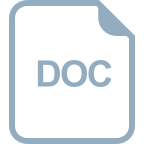