使用keil写一个51单片机猜拳游戏软件
时间: 2023-06-09 16:04:22 浏览: 109
好的,首先你需要了解猜拳游戏的规则,然后才能写出相应的程序。猜拳游戏是让两个玩家随机出拳,石头剪刀布分别用数字0、1、2表示,然后根据规则判断输赢,并输出结果。
以下是一个简单的示例程序,你可以根据自己的需求进行修改和完善:
```
#include <reg52.h>
#include <stdlib.h>
#include <time.h>
sbit LED1 = P1^0;
sbit LED2 = P1^1;
sbit LED3 = P1^2;
void delay(unsigned int x); // 延时函数
unsigned char getChoice(); // 获取玩家出拳
unsigned char getCpu(); // 获取CPU出拳
void judge(unsigned char player, unsigned char cpu); // 判断输赢
void main() {
unsigned char player, cpu;
unsigned char again;
srand(time(NULL)); // 初始化随机数种子
do {
player = getChoice();
cpu = getCpu();
judge(player, cpu);
// 等待重启游戏
LED1 = LED2 = LED3 = 0;
P0 = 0xff;
P1 = 0xff;
P2 = 0xff;
P3 = 0xff;
delay(1000);
LED1 = LED2 = LED3 = 1;
delay(1000);
LED1 = LED2 = LED3 = 0;
delay(1000);
LED1 = LED2 = LED3 = 1;
delay(1000);
LED1 = LED2 = LED3 = 0;
delay(1000);
// 询问是否再玩一次
do {
printf("\nPlay again? (y/n): ");
again = getchar();
fflush(stdin);
} while (again != 'y' && again != 'n');
} while (again == 'y');
}
unsigned char getChoice() {
unsigned char ch;
do {
printf("\nPlease choose (0 for Rock, 1 for Scissors, 2 for Paper): ");
ch = getchar();
fflush(stdin);
} while (ch < '0' || ch > '2');
return ch - '0';
}
unsigned char getCpu() {
return rand() % 3;
}
void judge(unsigned char player, unsigned char cpu) {
LED1 = LED2 = LED3 = 0;
switch (player) {
case 0: // Rock
P0 = 0xfe;
break;
case 1: // Scissors
P0 = 0xfd;
break;
case 2: // Paper
P0 = 0xfb;
break;
default:
break;
}
switch (cpu) {
case 0: // Rock
P1 = 0xfe;
break;
case 1: // Scissors
P1 = 0xfd;
break;
case 2: // Paper
P1 = 0xfb;
break;
default:
break;
}
if (player == cpu) { // 平局
LED1 = 1;
LED2 = 1;
LED3 = 1;
} else if (player == 0 && cpu == 1 || player == 1 && cpu == 2 || player == 2 && cpu == 0) { // 玩家赢
LED1 = 1;
} else { // CPU赢
LED3 = 1;
}
}
void delay(unsigned int x) {
unsigned int i, j;
for (i = 0; i < x; i++) {
for (j = 0; j < 120; j++) {
}
}
}
```
这个程序中,我们使用了51单片机的GPIO引脚控制LED灯,通过矩阵键盘获取用户的选择,同时使用了随机数函数获取CPU的出拳,最后再根据规则进行比较和输出结果。
相关推荐
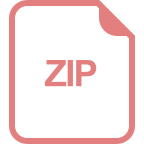
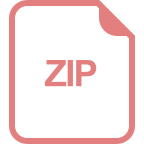













