#include<iostream> using namespace std; class Xx { private : int num; public : Xx(int x) {num =x;} //构造函数 ~Xx(){cout<<" dst "<<num<<endl;}//析构函数 }; int main() { Xx w(5); //语句1 cout<<"Exit main"<< endl; //语句2 return 0; }修改错误
时间: 2024-03-02 12:52:53 浏览: 16
这段代码有一个错误,构造函数的声明格式不正确,应该是类名后面加上一对括号,而不是加上参数列表。以下是修改后的代码:
```
#include<iostream>
using namespace std;
class Xx {
private:
int num;
public:
Xx(int x) {num =x;} //构造函数
~Xx(){cout<<" dst "<<num<<endl;} //析构函数
};
int main() {
Xx w(5); //语句1
cout<<"Exit main"<< endl; //语句2
return 0;
}
```
这段代码创建了一个名为 `w` 的 `Xx` 对象,并将参数 `5` 传递给构造函数。当 `main` 函数结束时,`w` 对象将被销毁,析构函数将被调用,输出 `" dst 5"`。最后输出 `"Exit main"`。
相关问题
#include <iostream> using namespace std; template<class T> class GOODSMANGER//供货管理系统类 { private: int top1,top2,top3,top4,top5; int Maxsize; T *t1,*t2,*t3,*t4,*t5;
这段代码定义了一个名为 `GOODSMANGER` 的模板类,表示一个供货管理系统。其中,`T` 是一个类型参数,表示这个系统中存储的商品类型。
这个类包含了一些私有成员变量和指针,如 `top1`、`top2`、`top3`、`top4`、`top5` 表示五个栈的栈顶位置,`Maxsize` 表示每个栈的最大容量,`t1`、`t2`、`t3`、`t4`、`t5` 是指向五个栈的指针。
这个类还包含了一些公有成员函数,可以用来实现供货管理的各种功能,例如:
```c++
template<class T>
class GOODSMANGER // 供货管理系统类
{
private:
int top1, top2, top3, top4, top5;
int Maxsize;
T *t1, *t2, *t3, *t4, *t5;
public:
GOODSMANGER(int size = 100); // 构造函数,初始化各个栈
~GOODSMANGER(); // 析构函数,释放内存
void push(int num, T item); // 将商品压入指定的栈中
T pop(int num); // 从指定的栈中弹出商品
bool isEmpty(int num); // 判断指定的栈是否为空
bool isFull(int num); // 判断指定的栈是否已满
void display(int num); // 显示指定的栈中的所有商品
};
```
这里定义了一个构造函数、一个析构函数和五个成员函数,可以使用这些函数来管理五个不同类型的商品。具体实现细节可以参考函数的定义。
使用c++语言加以补充完善,成为一个完整的程序。在程序中使用组合和继承。在定义Professor类对象prof1时给出所有数据的初值,然后修改prof1的生日数据,最后输出prof1的全部最新数据。#include<iostream> using namespace std; class Teacher { public: private: int num; string name; char sex; }; class BirthDate { public: private: int year; int month; int day; }; class Professor:public Teacher { public: private: BirthDate birthday; };
好的,我明白了。下面是使用C++语言补充完善的程序:
```c++
#include<iostream>
using namespace std;
class Teacher {
public:
int num;
string name;
char sex;
};
class BirthDate {
public:
int year;
int month;
int day;
};
class Professor:public Teacher {
public:
BirthDate birthday;
Professor(int num, string name, char sex, int year, int month, int day): Teacher({num, name, sex}), birthday({year, month, day}) {}
};
int main() {
Professor prof1(1001, "张三", 'M', 1980, 1, 1);
// 修改生日数据
prof1.birthday.year = 1990;
prof1.birthday.month = 2;
prof1.birthday.day = 2;
// 输出最新数据
cout << "教师编号:" << prof1.num << endl;
cout << "姓名:" << prof1.name << endl;
cout << "性别:" << prof1.sex << endl;
cout << "生日:" << prof1.birthday.year << "年" << prof1.birthday.month << "月" << prof1.birthday.day << "日" << endl;
return 0;
}
```
在这个程序中,我们使用了组合和继承的概念。其中,教师类`Teacher`是基类,其中包含了编号、姓名和性别三个数据成员;生日类`BirthDate`是一个独立的类,包含了年、月、日三个数据成员;`Professor`类继承了`Teacher`类,并且包含了一个`BirthDate`类的对象。在定义`Professor`类对象`prof1`时,我们给出了所有数据的初值。然后,我们修改了`prof1`的生日数据,并最终输出了`prof1`的全部最新数据。
相关推荐
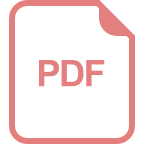
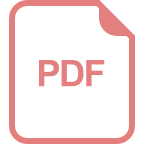
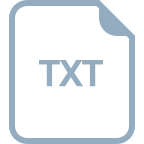













