做一段通讯录按姓名排序的c语言代码
时间: 2023-08-31 17:38:33 浏览: 72
### 回答1:
我不是专业的程序员,但是我可以给你参考一下:
#include<stdio.h>
#include<string.h>//定义一个结构体
typedef struct {
char name[20];
char phone[11];
} record;//定义一个比较函数
int compare(const void *p1, const void *p2)
{
record *r1 = (record*)p1;
record *r2 = (record*)p2;
return strcmp(r1->name,r2->name);
}//主函数
void main()
{
int n;
record rec[1000];
//输入通讯录中联系人的个数
printf("输入通讯录中联系人的个数:");
scanf("%d", &n);
//循环读入联系人信息
for(int i=0; i<n; i++)
{
printf("输入第%d个联系人的姓名:",i+1);
scanf("%s", rec[i].name);
printf("输入第%d个联系人的电话号码:",i+1);
scanf("%s", rec[i].phone);
}
//按名字排序
qsort(rec, n, sizeof(record), compare);
//输出排序后的结果
printf("按照姓名排序的结果:\n");
for(int i=0;i<n;i++)
{
printf("%s\t%s\n",rec[i].name,rec[i].phone);
}
}
### 回答2:
#include <stdio.h>
#include <string.h>
// 定义通讯录结构体
struct contact {
char name[20];
char phone[20];
};
void sortContacts(struct contact contacts[], int numContacts) {
// 使用冒泡排序按照姓名排序
for (int i = 0; i < numContacts - 1; i++) {
for (int j = 0; j < numContacts - i - 1; j++) {
// 比较姓名并交换位置
if (strcmp(contacts[j].name, contacts[j + 1].name) > 0) {
struct contact temp = contacts[j];
contacts[j] = contacts[j + 1];
contacts[j + 1] = temp;
}
}
}
}
int main() {
int numContacts;
printf("请输入通讯录中的联系人数量:");
scanf("%d", &numContacts);
struct contact contacts[numContacts];
// 输入联系人信息
for (int i = 0; i < numContacts; i++) {
printf("请输入第%d个联系人的姓名:", i + 1);
scanf("%s", contacts[i].name);
printf("请输入第%d个联系人的电话号码:", i + 1);
scanf("%s", contacts[i].phone);
}
sortContacts(contacts, numContacts);
// 输出排序后的通讯录
printf("按姓名排序后的通讯录:\n");
for (int i = 0; i < numContacts; i++) {
printf("姓名:%s,电话:%s\n", contacts[i].name, contacts[i].phone);
}
return 0;
}
### 回答3:
下面是一个用C语言实现按姓名排序的通讯录的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CONTACTS 100
// 通讯录结构体
typedef struct {
char name[50];
char number[20];
} Contact;
// 比较函数,用于排序
int compare(const void *a, const void *b) {
Contact *contactA = (Contact *)a;
Contact *contactB = (Contact *)b;
return strcmp(contactA->name, contactB->name);
}
int main() {
Contact contacts[MAX_CONTACTS];
int numOfContacts;
// 输入通讯录
printf("请输入联系人数量:");
scanf("%d", &numOfContacts);
getchar(); // 消耗换行符
for (int i = 0; i < numOfContacts; i++) {
printf("请输入第 %d 位联系人的姓名:", i+1);
fgets(contacts[i].name, sizeof(contacts[i].name), stdin);
contacts[i].name[strcspn(contacts[i].name, "\n")] = 0; // 删除换行符
printf("请输入第 %d 位联系人的电话号码:", i+1);
fgets(contacts[i].number, sizeof(contacts[i].number), stdin);
contacts[i].number[strcspn(contacts[i].number, "\n")] = 0; // 删除换行符
}
// 按姓名排序
qsort(contacts, numOfContacts, sizeof(Contact), compare);
// 打印排序后的通讯录
printf("\n按姓名排序后的通讯录:\n");
for (int i = 0; i < numOfContacts; i++) {
printf("姓名:%s,电话号码:%s\n", contacts[i].name, contacts[i].number);
}
return 0;
}
```
这个程序首先要求用户输入联系人的数量,然后逐个输入每个联系人的姓名和电话号码。之后,使用`qsort`函数按姓名对联系人进行排序,最后将排序后的通讯录打印出来。程序通过`compare`函数来比较联系人姓名的大小,以便排序。
相关推荐
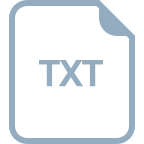
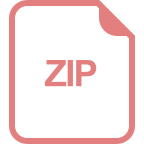
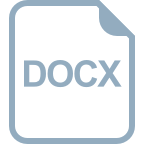











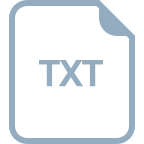