python thread join
时间: 2023-04-25 19:01:53 浏览: 81
Python中的线程join()方法是用来等待线程结束的。当一个线程调用join()方法时,主线程会等待该线程执行完毕后再继续执行。如果不调用join()方法,主线程会立即结束,而子线程可能还在执行中。因此,join()方法可以保证子线程执行完毕后再结束程序,避免出现意外的情况。
相关问题
python thread join函数
Python中的`join()`函数是用于等待线程执行完毕的方法。当调用线程的`join()`方法时,它会阻塞当前线程,直到被调用的线程执行完毕。
`join()`函数的语法如下:
```python
thread.join(timeout)
```
其中,`thread`是要等待的线程对象,`timeout`是可选参数,表示最长等待时间(以秒为单位)。如果不指定`timeout`,则`join()`方法会一直阻塞当前线程,直到被调用的线程执行完毕。
如果指定了`timeout`参数,那么`join()`方法会阻塞当前线程,最多等待`timeout`秒。如果在指定的时间内被调用的线程没有执行完毕,当前线程会继续执行。
`join()`方法常用于主线程等待子线程执行完毕,以确保所有线程都已经完成任务后再继续执行后续代码。
python thread join的用法
Python中的thread join方法用于等待线程结束。它会阻塞当前线程,直到调用join方法的线程结束运行并退出。可以使用join方法来确保在主线程结束之前,所有的子线程都已经结束运行。
join方法有一个可选参数timeout,它指定了最长的等待时间。如果超过了这个时间,join方法会退出并返回。如果没有指定timeout参数,则join方法会一直等待到线程结束为止。
以下是一个示例代码:
```
import threading
import time
def worker():
print("Worker thread started")
time.sleep(5)
print("Worker thread finished")
t = threading.Thread(target=worker)
t.start()
print("Main thread started")
t.join()
print("Main thread finished")
```
输出:
```
Main thread started
Worker thread started
Worker thread finished
Main thread finished
```
在这个例子中,主线程启动一个工作线程,并等待它完成。join方法会阻塞主线程,直到工作线程结束运行并退出。在这个例子中,工作线程会等待5秒钟才退出。在主线程等待期间,它会打印出“Main thread started”和“Main thread finished”消息。在工作线程运行期间,它会打印出“Worker thread started”和“Worker thread finished”消息。
相关推荐
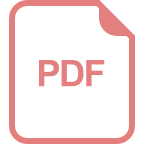
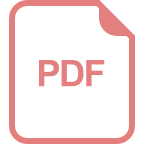
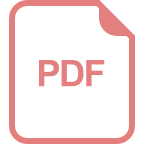












