#include <stdio.h> #define PI 3.1415926 // 定义圆周率为常数 float calc_area(float radius); // 计算圆的面积 float calc_circumference(float radius); // 计算圆的周长 void display(float area, float circumference); // 显示圆的面积和周长 int main() { float radius; printf("请输入圆的半径:"); scanf("%f", &radius); float area = calc_area(radius); float circumference = calc_circumference(radius); display(area, circumference); return 0; } float calc_area(float radius) { return PI * radius * radius; } float calc_circumference(float radius) { return 2 * PI * radius; } void display(float area, float circumference) { printf("圆的面积为:%f\n", area); printf("圆的周长为:%f\n", circumference); }请帮我把这个程序的命名全换成中文
时间: 2023-07-15 21:12:51 浏览: 78
#include <stdio.h>
#define 圆周率 3.1415926 // 定义圆周率为常数
float 计算面积(float 半径); // 计算圆的面积
float 计算周长(float 半径); // 计算圆的周长
void 显示(float 面积, float 周长); // 显示圆的面积和周长
int main() {
float 半径;
printf("请输入圆的半径:");
scanf("%f", &半径);
float 面积 = 计算面积(半径);
float 周长 = 计算周长(半径);
显示(面积, 周长);
return 0;
}
float 计算面积(float 半径) {
return 圆周率 * 半径 * 半径;
}
float 计算周长(float 半径) {
return 2 * 圆周率 * 半径;
}
void 显示(float 面积, float 周长) {
printf("圆的面积为:%f\n", 面积);
printf("圆的周长为:%f\n", 周长);
}
相关问题
#include<stdio.h> //begin-- SaSs #define PI 3.14; int mian() F float r;area; scanf("%f" &r); 2 area-
这个代码片段似乎包含了一些拼写错误和格式问题,并不是一个完整的程序。从这部分看,它像是试图编写一个计算圆面积的C语言程序。首先,`#define PI 3.14;` 定义了一个常量PI用于圆周率计算。然后有一个函数声明部分:
```c
int main() {
```
但主体部分有几处问题:
1. `mian()` 应该是 `main()`,函数名首字母应大写。
2. 变量 `area` 没有初始化并且应该在主函数中声明。
3. `scanf` 后面的分号应该是逗号,用于输入半径并赋值给变量 `r`。
4. 缺少对输入半径后的计算和输出面积的部分。
正确的代码可能是这样的:
```c
#include<stdio.h>
#define PI 3.14
int main() {
float radius;
float area;
scanf("%f", &radius);
area = PI * radius * radius;
printf("The area of the circle with radius %.2f is %.2f\n", radius, area);
return 0;
}
```
用c语言编写程序输入圆的半径,求圆的周长和面积。 要求定义圆周率为如下宏常量 #define PI 3.14159结果保留两位小数
下面是用C语言编写输入圆的半径,求圆的周长和面积的程序:
```
#include <stdio.h>
#define PI 3.1415926 // 宏常量
int main()
{
float radius; // 圆的半径
float perimeter; // 圆的周长
float area; // 圆的面积
printf("请输入圆的半径:");
scanf("%f", &radius);
perimeter = 2 * PI * radius;
area = PI * radius * radius;
printf("圆的周长为:%f\n", perimeter);
printf("圆的面积为:%f\n", area);
return 0;
}
```
其中,定义了一个宏常量PI,用来表示圆周率。在程序中,使用用户输入的半径和圆周率计算圆的周长和面积,并输出结果。
阅读全文
相关推荐
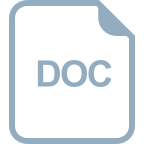
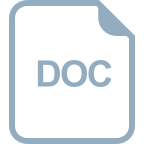
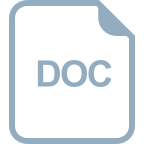
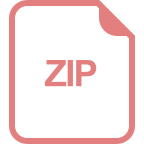
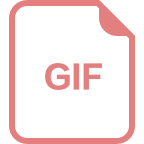
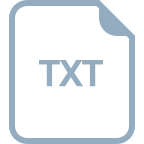
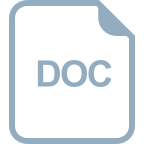
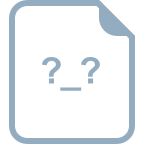
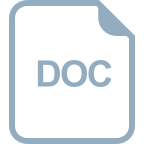
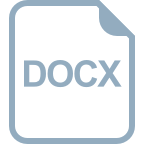
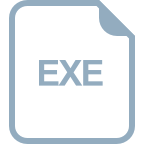
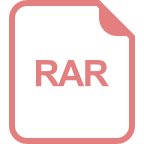
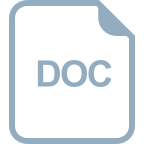
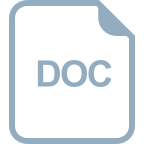