请写一个函数,满足一下功能,对于给定的串链表,用户输入字符串,要求统计字符串在串链表中出现的次数
时间: 2024-03-15 10:47:46 浏览: 55
好的,我可以帮你写一个函数来实现这个功能。首先,我们需要定义一个结构体来表示链表中的每个节点,包括一个指向下一个节点的指针和一个字符串成员来存储字符串。代码如下:
```c
typedef struct Node {
char* str;
struct Node* next;
} Node;
```
接下来,我们可以编写一个函数来创建这个链表,读取用户输入并将输入的字符串插入到链表中:
```c
Node* createList() {
Node* head = NULL;
Node* tail = NULL;
char input[100];
while (1) {
printf("请输入一个字符串(输入exit结束):\n");
scanf("%s", input);
if (strcmp(input, "exit") == 0) {
break;
}
Node* node = (Node*) malloc(sizeof(Node));
node->str = (char*) malloc(strlen(input) + 1);
strcpy(node->str, input);
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
```
这个函数会一直读取用户输入,直到用户输入“exit”为止。每次读取到一个字符串,就会创建一个新的节点,并将节点插入到链表的尾部。
接下来,我们可以编写一个函数来统计给定字符串在链表中出现的次数:
```c
int countOccurrences(Node* head, char* str) {
int count = 0;
Node* current = head;
while (current != NULL) {
if (strcmp(current->str, str) == 0) {
count++;
}
current = current->next;
}
return count;
}
```
这个函数会遍历整个链表,每当遇到与给定字符串相同时,计数器就会加1。最后,函数将计数器的值作为结果返回。
最后,我们可以编写一个主函数来调用这些函数并输出结果:
```c
int main() {
Node* head = createList();
char input[100];
while (1) {
printf("请输入要查询的字符串(输入exit结束):\n");
scanf("%s", input);
if (strcmp(input, "exit") == 0) {
break;
}
int count = countOccurrences(head, input);
printf("'%s' 在链表中出现了 %d 次\n", input, count);
}
return 0;
}
```
这个主函数会先调用 createList 函数来创建链表,然后不断读取用户输入的字符串,并调用 countOccurrences 函数来统计字符串在链表中出现的次数,最后输出结果。
阅读全文
相关推荐
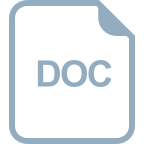
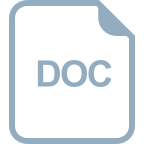
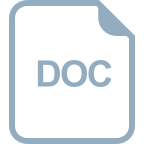
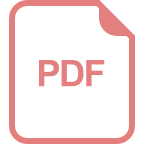
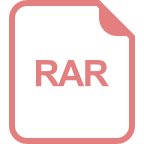
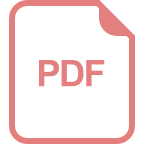
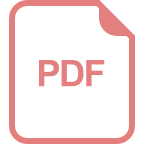
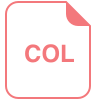






