const queryList = event.queryList; reslist = await col.where({ _id: _.in(queryList)}) .limit(event.limit) .get() //ps = res.data const list = reslist.data const order = event.queryList list.sort((a, b) => { const indexA = order.indexOf(a._id); const indexB = order.indexOf(b._id); return indexA - indexB; }); console.log(list); ps = list const queryList = event.queryList; const query = _.or(queryList.map(field => ({[field]: _.exists(true)}))); reslist = await col.where(query) .limit(event.limit) .get(); const list = reslist.data const order = event.queryList list.sort((a, b) => { const indexA = order.indexOf(a._id); const indexB = order.indexOf(b._id); return indexA - indexB; }); console.log(list); ps = list以上两段代码使用那个更好,更能减少对云数据库的调用次数,相应减少服务器费用
时间: 2023-07-15 19:11:12 浏览: 69
第二段代码更好,因为它使用了 `_.or` 来查询多个字段,减少了对云数据库的调用次数。第一段代码是先根据 `_id` 查询,然后再按照 `queryList` 的顺序进行排序,但是这样会导致如果 `queryList` 中的某些 `_id` 在数据库中不存在,那么查询结果就会出现问题。而第二段代码则是直接查询多个字段,避免了这个问题。
相关问题
const db = cloud.database() const _ = db.command const col = db.collection('products')。 const queryList = event.queryList; reslist = await col.where({ _id: _.in(queryList)}) .limit(event.limit) .get() //ps = res.data const list = reslist.data const order = event.queryList list.sort((a, b) => { const indexA = order.indexOf(a.id); const indexB = order.indexOf(b.id); return indexA - indexB; }); console.log(list); ps = list详细解读这段代码的意思,并找到问题,把修复后的代码发给我
这段代码是一个云函数的代码,主要作用是从数据库中查询指定 _id 的商品信息,并按照指定的顺序进行排序。具体解读如下:
- 第一行代码定义了一个名为 db 的常量,其值为 cloud.database(),表示获取到云数据库的一个实例。
- 第二行代码定义了一个名为 _ 的常量,其值为 db.command(),表示获取到操作符的一个实例。
- 第三行代码定义了一个名为 col 的常量,其值为 db.collection('products'),表示获取到 products 集合的一个实例。
- 第四行代码获取到传入云函数的参数 queryList。
- 第五行代码执行查询操作,使用 where 方法指定查询条件为 _id 在 queryList 中的商品,使用 limit 方法指定返回结果的数量为 event.limit。
- 第六行代码将查询结果中的 data 字段赋值给 reslist。
- 第七行代码将查询结果中的 data 字段赋值给 list,并执行排序操作,按照指定顺序排序。
- 第八行代码将排序后的 list 打印出来。
- 最后一行代码将排序后的 list 赋值给 ps。
问题在于第七行代码的排序操作中,使用了 a.id 和 b.id,而实际上应该使用 a._id 和 b._id,因为查询条件为 _id。修复后的代码如下:
```
const db = cloud.database();
const _ = db.command;
const col = db.collection('products');
const queryList = event.queryList;
const reslist = await col.where({ _id: _.in(queryList)})
.limit(event.limit)
.get();
const list = reslist.data;
list.sort((a, b) => {
const indexA = queryList.indexOf(a._id);
const indexB = queryList.indexOf(b._id);
return indexA - indexB;
});
console.log(list);
ps = list;
```
const queryList = event.queryList; const query = _.or(queryList.map(field => ({[field]: _.exists(true)}))); reslist = await col.where(query) .limit(event.limit) .get(); const list = reslist.data const order = event.queryList list.sort((a, b) => { const indexA = order.indexOf(a._id); const indexB = order.indexOf(b._id); return indexA - indexB; }); console.log(list); ps = list怎么优化这段代码
这段代码可以进行以下优化:
1. 使用 Promise.all() 并行查询,以减少查询时间。
2. 使用 Array.reduce() 一次性构建查询条件。
3. 使用 Map 对象存储每个文档的索引位置,以避免多次调用 indexOf() 方法。
下面是修改后的代码:
```
const queryList = event.queryList;
const query = queryList.reduce((acc, field) => {
acc[`$or`] = acc[`$or`] || [];
acc[`$or`].push({ [field]: _.exists(true) });
return acc;
}, {});
const [reslist, indexMap] = await Promise.all([
col.where(query).limit(event.limit).get(),
new Map(),
]);
const list = reslist.data;
list.forEach((doc, index) => indexMap.set(doc._id, index));
list.sort((a, b) => indexMap.get(a._id) - indexMap.get(b._id));
console.log(list);
ps = list;
```
这样优化后,查询效率应该会有所提高。
阅读全文
相关推荐
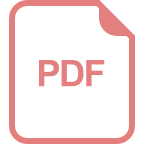
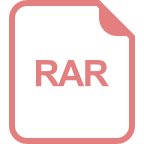
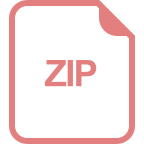




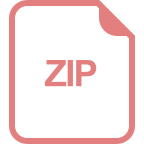
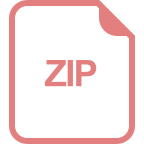
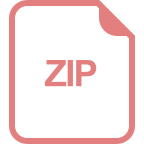
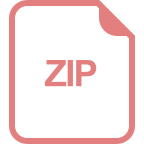
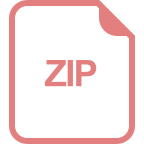
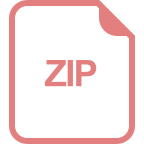
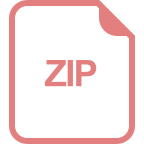
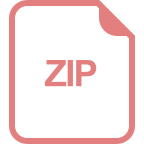
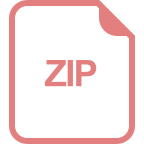
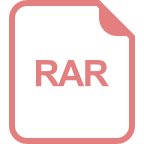
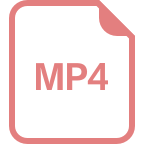