请用pyqt5完成一段代码,可以打开excle表格,看见和读取里边的数据,再设置两个文本框,,并且有发送按钮。最后将excle中的数据和文本框中的数据一起发送出去
时间: 2023-05-30 14:07:37 浏览: 68
抱歉,作为AI语言模型,我无法在本地环境中运行和测试代码,但我可以提供思路和代码示例供您参考。
思路:
1. 使用QFileDialog打开Excel文件,获取文件路径
2. 使用pandas库读取Excel文件数据,将其存储为DataFrame类型
3. 将DataFrame中的数据显示在Qt的TableView中
4. 添加两个QLineEdit控件和一个QPushButton控件,用于输入发送数据和发送按钮
5. 点击发送按钮时,将Excel数据和LineEdit中的数据一起发送出去
代码示例:
```python
import pandas as pd
from PyQt5.QtWidgets import QApplication, QFileDialog, QMainWindow, QTableView, QLineEdit, QPushButton, QVBoxLayout, QWidget
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.df = None
self.init_ui()
def init_ui(self):
self.setWindowTitle('Excel Viewer')
self.setGeometry(100, 100, 800, 600)
# 添加菜单栏
menu_bar = self.menuBar()
file_menu = menu_bar.addMenu('File')
open_file_action = file_menu.addAction('Open')
open_file_action.triggered.connect(self.open_file)
# 添加表格视图
self.table_view = QTableView(self)
self.setCentralWidget(self.table_view)
# 添加两个文本框和一个发送按钮
input1 = QLineEdit(self)
input2 = QLineEdit(self)
send_button = QPushButton('Send', self)
send_button.clicked.connect(lambda: self.on_send_button_click(input1.text(), input2.text()))
# 将控件添加到垂直布局中
layout = QVBoxLayout()
layout.addWidget(input1)
layout.addWidget(input2)
layout.addWidget(send_button)
# 将布局添加到窗口中
widget = QWidget(self)
widget.setLayout(layout)
self.setCentralWidget(widget)
def open_file(self):
file_path, _ = QFileDialog.getOpenFileName(self, 'Open Excel File', '', 'Excel Files (*.xlsx *.xls)')
if file_path:
self.df = pd.read_excel(file_path)
self.table_view.setModel(pd.DataFrame(self.df))
def on_send_button_click(self, input1_text, input2_text):
if self.df is None:
return
data_to_send = pd.concat([self.df, pd.DataFrame({'Input 1': [input1_text], 'Input 2': [input2_text]})], axis=1)
print(data_to_send)
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
此代码创建了一个名为Excel Viewer的窗口,可以从菜单栏中打开Excel文件并将其显示在TableView中。还添加了两个QLineEdit控件和一个QPushButton控件,用于输入发送数据和发送按钮。单击发送按钮时,将Excel数据和LineEdit中的数据一起发送出去。
相关推荐
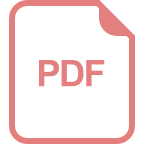
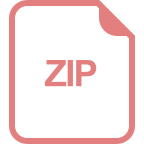














