请根据以下要求用c++编写程序:为日期定义一个类。属性为年、月、日。要求重载 “++”、“--”(自增和自减运算符要求分前置和后置)、“<<”、“>>”等运算符,实现今天日期的输入输出、明天、昨天的日期求解和输出。
时间: 2023-12-24 21:03:38 浏览: 146
好的,以下是我为您编写的代码,希望对您有所帮助:
```c++
#include <iostream>
using namespace std;
class Date {
private:
int year;
int month;
int day;
public:
Date(int y = 0, int m = 0, int d = 0) : year(y), month(m), day(d) {} // 构造函数
void setDate(int y, int m, int d) { // 设置日期
year = y;
month = m;
day = d;
}
int getYear() const { return year; } // 获取年份
int getMonth() const { return month; } // 获取月份
int getDay() const { return day; } // 获取日份
// 重载自增运算符
Date operator++() {
int daysInMonth = 31;
if (month == 4 || month == 6 || month == 9 || month == 11) daysInMonth = 30;
else if (month == 2) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) daysInMonth = 29;
else daysInMonth = 28;
}
day++;
if (day > daysInMonth) {
day = 1;
month++;
if (month > 12) {
month = 1;
year++;
}
}
return *this;
}
// 重载自减运算符
Date operator--() {
day--;
if (day < 1) {
month--;
if (month < 1) {
month = 12;
year--;
}
int daysInMonth = 31;
if (month == 4 || month == 6 || month == 9 || month == 11) daysInMonth = 30;
else if (month == 2) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) daysInMonth = 29;
else daysInMonth = 28;
}
day = daysInMonth;
}
return *this;
}
// 重载后置自增运算符
Date operator++(int) {
Date temp = *this;
++(*this);
return temp;
}
// 重载后置自减运算符
Date operator--(int) {
Date temp = *this;
--(*this);
return temp;
}
// 重载输出运算符
friend ostream& operator<<(ostream& os, const Date& date) {
os << date.year << "-" << date.month << "-" << date.day;
return os;
}
// 重载输入运算符
friend istream& operator>>(istream& is, Date& date) {
is >> date.year >> date.month >> date.day;
return is;
}
};
int main() {
Date today;
cout << "请输入今天的日期(格式:年 月 日):";
cin >> today;
cout << "今天的日期为:" << today << endl;
cout << "明天的日期为:" << ++today << endl;
cout << "昨天的日期为:" << --today << endl;
return 0;
}
```
上述代码中,我们定义了一个名为 `Date` 的类,用于表示日期数据。该类包含了年、月、日等属性,以及设置日期、获取日期、自增自减、输入输出等方法。
在 `main` 函数中,我们通过用户输入来获取今天的日期,并输出今天、明天、昨天的日期,具体实现使用了类中重载的自增自减运算符。
希望这个程序对您有所帮助!
阅读全文
相关推荐
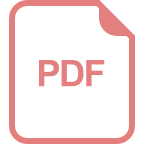
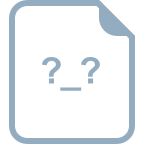


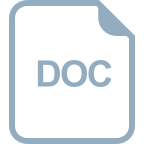
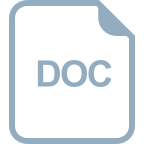
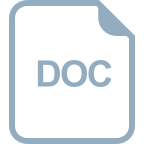
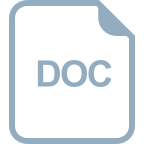
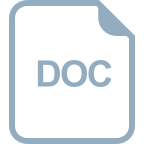
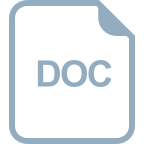
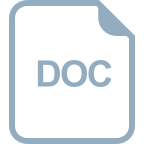
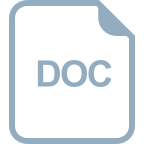
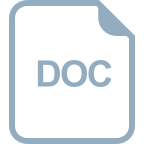
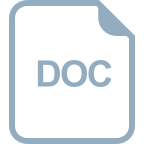
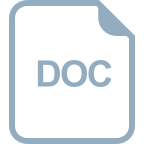
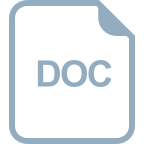

