国密算法(SM2-SM3)Java 使用 demo maven 工程
时间: 2024-01-24 12:02:48 浏览: 50
很高兴回答你的问题!以下是一个使用国密算法(SM2-SM3)的Java Demo Maven工程,供您参考:
1. 首先,在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>com.huawei.security</groupId>
<artifactId>sm</artifactId>
<version>2.0.5.300</version>
</dependency>
```
2. 创建SM2密钥对
```java
import com.huawei.security.sm.SM2KeyPair;
import com.huawei.security.sm.SM2PrivateKey;
import com.huawei.security.sm.SM2PublicKey;
import java.security.NoSuchAlgorithmException;
import java.security.NoSuchProviderException;
import java.security.Security;
public class SM2Demo {
public static void main(String[] args) throws NoSuchProviderException, NoSuchAlgorithmException {
Security.addProvider(new org.bouncycastle.jce.provider.BouncyCastleProvider());
SM2KeyPair sm2KeyPair = new SM2KeyPair();
SM2PublicKey publicKey = sm2KeyPair.getPublicKey();
SM2PrivateKey privateKey = sm2KeyPair.getPrivateKey();
System.out.println("publicKey: " + publicKey.getHexString());
System.out.println("privateKey: " + privateKey.getHexString());
}
}
```
3. SM2加密和解密
```java
import com.huawei.security.sm.SM2PrivateKey;
import com.huawei.security.sm.SM2PublicKey;
import com.huawei.security.sm.SM2Engine;
import java.security.NoSuchAlgorithmException;
import java.security.NoSuchProviderException;
import java.security.Security;
import java.security.InvalidAlgorithmParameterException;
import java.security.InvalidKeyException;
import java.security.KeyPairGenerator;
import javax.crypto.Cipher;
import javax.crypto.NoSuchPaddingException;
public class SM2Demo {
public static void main(String[] args) throws NoSuchProviderException, NoSuchAlgorithmException, InvalidAlgorithmParameterException, InvalidKeyException, NoSuchPaddingException {
Security.addProvider(new org.bouncycastle.jce.provider.BouncyCastleProvider());
// 创建密钥对
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("EC", "BC");
keyPairGenerator.initialize(256);
SM2KeyPair sm2KeyPair = new SM2KeyPair(keyPairGenerator.generateKeyPair());
// 获取公钥和私钥
SM2PublicKey publicKey = sm2KeyPair.getPublicKey();
SM2PrivateKey privateKey = sm2KeyPair.getPrivateKey();
// 加密
SM2Engine sm2Engine = new SM2Engine();
Cipher cipher = Cipher.getInstance(sm2Engine.getAlgorithmName(), "BC");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] plaintext = "Hello, world!".getBytes();
byte[] ciphertext = cipher.doFinal(plaintext);
System.out.println("ciphertext: " + new String(ciphertext));
// 解密
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decrypted = cipher.doFinal(ciphertext);
System.out.println("decrypted: " + new String(decrypted));
}
}
```
4. SM3哈希算法
```java
import com.huawei.security.sm.SM3Digest;
import java.security.NoSuchAlgorithmException;
import java.security.NoSuchProviderException;
import java.security.Security;
public class SM3Demo {
public static void main(String[] args) throws NoSuchProviderException, NoSuchAlgorithmException {
Security.addProvider(new org.bouncycastle.jce.provider.BouncyCastleProvider());
// 计算哈希值
SM3Digest sm3Digest = new SM3Digest();
byte[] plaintext = "Hello, world!".getBytes();
sm3Digest.update(plaintext, 0, plaintext.length);
byte[] hash = new byte[sm3Digest.getDigestSize()];
sm3Digest.doFinal(hash, 0);
System.out.println("hash: " + org.bouncycastle.util.encoders.Hex.toHexString(hash));
}
}
```
希望这个Demo工程能够帮助您使用国密算法(SM2-SM3)的Java开发。如果您有任何疑问,请随时联系我!
相关推荐
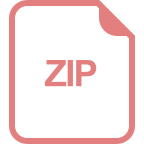
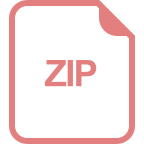














