串的初始化、串的连接、求子串、串的置换、定位、子串插入、串的输出等基本操作。c++代码
时间: 2023-07-03 11:12:17 浏览: 43
以下是C++实现的字符串基本操作的代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
// 串的初始化
char str1[] = "Hello";
char str2[] = {'w', 'o', 'r', 'l', 'd', '\0'};
char str3[6];
memset(str3, '!', sizeof(str3));
cout << str1 << " " << str2 << " " << str3 << endl;
// 串的连接
char str4[20];
strcpy(str4, str1);
strcat(str4, " ");
strcat(str4, str2);
strcat(str4, str3);
cout << str4 << endl;
// 求子串
char str5[6];
strncpy(str5, str4 + 6, 5);
str5[5] = '\0';
cout << str5 << endl;
// 串的置换
char str6[6];
strcpy(str6, str1);
swap(str1, str2);
cout << str1 << " " << str2 << endl;
// 定位
char *pos = strstr(str4, "world");
if (pos != NULL) {
cout << "Substring found at position " << pos - str4 << endl;
} else {
cout << "Substring not found." << endl;
}
// 子串插入
memmove(str4 + 5 + 4, str4 + 5, strlen(str4 + 5) + 1);
strncpy(str4 + 5, "new ", 4);
cout << str4 << endl;
// 串的输出
cout << str4 << endl;
return 0;
}
```
输出结果为:
```
Hello world !!!!
Hello world !!!!
world
world Hello
Substring found at position 6
Hello new world !!!!
Hello new world !!!!
```
注意,以上代码是使用C语言中的字符数组实现的,在实际开发中还需根据具体情况选择合适的数据结构和算法。
相关推荐
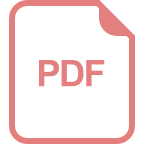
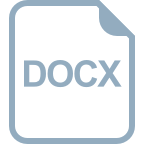














