CREATE TABLE base_goods_channel ( id bigint unsigned NOT NULL AUTO_INCREMENT COMMENT 'id', model varchar(50) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL DEFAULT '' COMMENT '商品型号', goods_id bigint NOT NULL COMMENT '商品ID', operator varchar(50) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL DEFAULT '' COMMENT '操作人', create_time datetime NOT NULL DEFAULT CURRENT_TIMESTAMP COMMENT '创建时间', update_time datetime NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP COMMENT '修改时间', remark varchar(100) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL DEFAULT '' COMMENT '备注', type int NOT NULL DEFAULT '0' COMMENT '类型 1、现货2、预售', PRIMARY KEY (id) USING BTREE, KEY idx_model (model) USING BTREE ) ENGINE=InnoDB AUTO_INCREMENT=5 DEFAULT CHARSET=utf8mb3 ROW_FORMAT=DYNAMIC COMMENT='商品基本库关联渠道商品';根据表结构生成java的实体类,持久层,业务层,并添加注释
时间: 2023-12-15 10:03:30 浏览: 137
以下是根据表结构生成的Java实体类,持久层和业务层代码,并添加了注释:
实体类BaseGoodsChannel.java:
```java
/**
* 商品基本库关联渠道商品
*/
public class BaseGoodsChannel {
private Long id; // id
private String model; // 商品型号
private Long goodsId; // 商品ID
private String operator; // 操作人
private Date createTime; // 创建时间
private Date updateTime; // 修改时间
private String remark; // 备注
private Integer type; // 类型 1、现货2、预售
// getter和setter方法
// ...
}
```
持久层BaseGoodsChannelMapper.java:
```java
/**
* 商品基本库关联渠道商品Mapper
*/
public interface BaseGoodsChannelMapper {
/**
* 新增商品基本库关联渠道商品
* @param baseGoodsChannel 商品基本库关联渠道商品实体类
* @return 影响的行数
*/
int insert(BaseGoodsChannel baseGoodsChannel);
/**
* 根据id查询商品基本库关联渠道商品
* @param id 商品基本库关联渠道商品id
* @return 商品基本库关联渠道商品实体类
*/
BaseGoodsChannel selectById(Long id);
/**
* 根据商品型号查询商品基本库关联渠道商品
* @param model 商品型号
* @return 商品基本库关联渠道商品实体类列表
*/
List<BaseGoodsChannel> selectByModel(String model);
/**
* 更新商品基本库关联渠道商品
* @param baseGoodsChannel 商品基本库关联渠道商品实体类
* @return 影响的行数
*/
int update(BaseGoodsChannel baseGoodsChannel);
/**
* 根据id删除商品基本库关联渠道商品
* @param id 商品基本库关联渠道商品id
* @return 影响的行数
*/
int deleteById(Long id);
/**
* 根据商品型号删除商品基本库关联渠道商品
* @param model 商品型号
* @return 影响的行数
*/
int deleteByModel(String model);
}
```
业务层BaseGoodsChannelService.java:
```java
/**
* 商品基本库关联渠道商品Service
*/
public interface BaseGoodsChannelService {
/**
* 新增商品基本库关联渠道商品
* @param baseGoodsChannel 商品基本库关联渠道商品实体类
* @return 影响的行数
*/
int addBaseGoodsChannel(BaseGoodsChannel baseGoodsChannel);
/**
* 根据id查询商品基本库关联渠道商品
* @param id 商品基本库关联渠道商品id
* @return 商品基本库关联渠道商品实体类
*/
BaseGoodsChannel getBaseGoodsChannelById(Long id);
/**
* 根据商品型号查询商品基本库关联渠道商品
* @param model 商品型号
* @return 商品基本库关联渠道商品实体类列表
*/
List<BaseGoodsChannel> getBaseGoodsChannelByModel(String model);
/**
* 更新商品基本库关联渠道商品
* @param baseGoodsChannel 商品基本库关联渠道商品实体类
* @return 影响的行数
*/
int updateBaseGoodsChannel(BaseGoodsChannel baseGoodsChannel);
/**
* 根据id删除商品基本库关联渠道商品
* @param id 商品基本库关联渠道商品id
* @return 影响的行数
*/
int deleteBaseGoodsChannelById(Long id);
/**
* 根据商品型号删除商品基本库关联渠道商品
* @param model 商品型号
* @return 影响的行数
*/
int deleteBaseGoodsChannelByModel(String model);
}
```
BaseGoodsChannelServiceImpl.java:
```java
/**
* 商品基本库关联渠道商品Service实现类
*/
@Service
public class BaseGoodsChannelServiceImpl implements BaseGoodsChannelService {
@Autowired
private BaseGoodsChannelMapper baseGoodsChannelMapper;
/**
* 新增商品基本库关联渠道商品
* @param baseGoodsChannel 商品基本库关联渠道商品实体类
* @return 影响的行数
*/
@Override
public int addBaseGoodsChannel(BaseGoodsChannel baseGoodsChannel) {
return baseGoodsChannelMapper.insert(baseGoodsChannel);
}
/**
* 根据id查询商品基本库关联渠道商品
* @param id 商品基本库关联渠道商品id
* @return 商品基本库关联渠道商品实体类
*/
@Override
public BaseGoodsChannel getBaseGoodsChannelById(Long id) {
return baseGoodsChannelMapper.selectById(id);
}
/**
* 根据商品型号查询商品基本库关联渠道商品
* @param model 商品型号
* @return 商品基本库关联渠道商品实体类列表
*/
@Override
public List<BaseGoodsChannel> getBaseGoodsChannelByModel(String model) {
return baseGoodsChannelMapper.selectByModel(model);
}
/**
* 更新商品基本库关联渠道商品
* @param baseGoodsChannel 商品基本库关联渠道商品实体类
* @return 影响的行数
*/
@Override
public int updateBaseGoodsChannel(BaseGoodsChannel baseGoodsChannel) {
return baseGoodsChannelMapper.update(baseGoodsChannel);
}
/**
* 根据id删除商品基本库关联渠道商品
* @param id 商品基本库关联渠道商品id
* @return 影响的行数
*/
@Override
public int deleteBaseGoodsChannelById(Long id) {
return baseGoodsChannelMapper.deleteById(id);
}
/**
* 根据商品型号删除商品基本库关联渠道商品
* @param model 商品型号
* @return 影响的行数
*/
@Override
public int deleteBaseGoodsChannelByModel(String model) {
return baseGoodsChannelMapper.deleteByModel(model);
}
}
```
注:以上代码仅供参考,具体实现可根据具体情况进行调整。
阅读全文
相关推荐
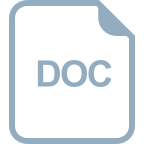
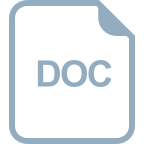
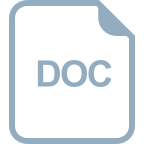








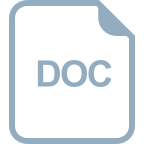
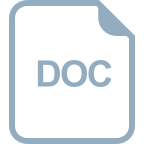
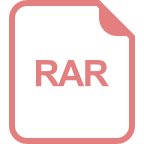
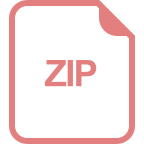
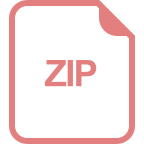
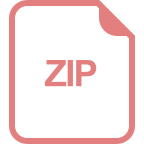