基于java的图书管理系统
时间: 2023-09-11 22:04:06 浏览: 77
一个基于Java的图书管理系统应该包括以下功能:
1. 图书信息管理:包括图书的添加、修改、删除和查询功能,以及图书分类的管理。
2. 借阅管理:包括借阅记录的添加、修改、删除和查询功能,以及借阅规则的设置。
3. 用户管理:包括读者信息的添加、修改、删除和查询功能,以及权限管理。
4. 统计报表:包括图书借阅统计、读者借阅统计等。
5. 系统设置:包括系统参数的设置、数据备份和恢复功能等。
下面是一个简单的图书管理系统的代码示例:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class BookManagementSystem {
// 图书类
static class Book {
private String name; // 书名
private String author; // 作者
private String category; // 分类
private boolean borrowed; // 是否被借阅
public Book(String name, String author, String category) {
this.name = name;
this.author = author;
this.category = category;
this.borrowed = false;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
public String getCategory() {
return category;
}
public boolean isBorrowed() {
return borrowed;
}
public void setBorrowed(boolean borrowed) {
this.borrowed = borrowed;
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", author='" + author + '\'' +
", category='" + category + '\'' +
", borrowed=" + borrowed +
'}';
}
}
// 图书管理类
static class BookManager {
private List<Book> books;
public BookManager() {
books = new ArrayList<>();
}
// 添加图书
public void addBook(Book book) {
books.add(book);
}
// 修改图书
public void updateBook(int index, Book book) {
books.set(index, book);
}
// 删除图书
public void removeBook(int index) {
books.remove(index);
}
// 查询图书
public List<Book> searchBook(String keyword) {
List<Book> result = new ArrayList<>();
for (Book book : books) {
if (book.getName().contains(keyword) ||
book.getAuthor().contains(keyword) ||
book.getCategory().contains(keyword)) {
result.add(book);
}
}
return result;
}
// 借阅图书
public boolean borrowBook(int index) {
Book book = books.get(index);
if (book.isBorrowed()) {
return false;
} else {
book.setBorrowed(true);
return true;
}
}
// 归还图书
public void returnBook(int index) {
Book book = books.get(index);
book.setBorrowed(false);
}
// 统计借阅图书数量
public int countBorrowedBooks() {
int count = 0;
for (Book book : books) {
if (book.isBorrowed()) {
count++;
}
}
return count;
}
// 统计图书数量
public int countBooks() {
return books.size();
}
// 打印所有图书信息
public void printAllBooks() {
System.out.println("所有图书信息:");
for (Book book : books) {
System.out.println(book);
}
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
BookManager bookManager = new BookManager();
// 添加几本图书
bookManager.addBook(new Book("Java程序设计", "张三", "计算机"));
bookManager.addBook(new Book("数据结构与算法", "李四", "计算机"));
bookManager.addBook(new Book("高等数学", "王五", "数学"));
// 主菜单
while (true) {
System.out.println("图书管理系统");
System.out.println("1. 添加图书");
System.out.println("2. 修改图书");
System.out.println("3. 删除图书");
System.out.println("4. 查询图书");
System.out.println("5. 借阅图书");
System.out.println("6. 归还图书");
System.out.println("7. 统计借阅图书数量");
System.out.println("8. 统计图书数量");
System.out.println("9. 打印所有图书信息");
System.out.println("0. 退出系统");
System.out.print("请输入选项:");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.print("请输入书名:");
String name = scanner.next();
System.out.print("请输入作者:");
String author = scanner.next();
System.out.print("请输入分类:");
String category = scanner.next();
bookManager.addBook(new Book(name, author, category));
break;
case 2:
System.out.print("请输入要修改的图书编号:");
int index2 = scanner.nextInt();
System.out.print("请输入书名:");
String name2 = scanner.next();
System.out.print("请输入作者:");
String author2 = scanner.next();
System.out.print("请输入分类:");
String category2 = scanner.next();
bookManager.updateBook(index2, new Book(name2, author2, category2));
break;
case 3:
System.out.print("请输入要删除的图书编号:");
int index3 = scanner.nextInt();
bookManager.removeBook(index3);
break;
case 4:
System.out.print("请输入关键字:");
String keyword = scanner.next();
List<Book> result = bookManager.searchBook(keyword);
System.out.println("查询结果:");
for (Book book : result) {
System.out.println(book);
}
break;
case 5:
System.out.print("请输入要借阅的图书编号:");
int index5 = scanner.nextInt();
boolean success = bookManager.borrowBook(index5);
if (success) {
System.out.println("借阅成功!");
} else {
System.out.println("借阅失败,该图书已被借阅!");
}
break;
case 6:
System.out.print("请输入要归还的图书编号:");
int index6 = scanner.nextInt();
bookManager.returnBook(index6);
System.out.println("归还成功!");
break;
case 7:
int count7 = bookManager.countBorrowedBooks();
System.out.println("借阅图书数量:" + count7);
break;
case 8:
int count8 = bookManager.countBooks();
System.out.println("图书数量:" + count8);
break;
case 9:
bookManager.printAllBooks();
break;
case 0:
System.out.println("感谢使用!");
return;
default:
System.out.println("无效选项,请重新输入!");
}
System.out.println();
}
}
}
```
请注意,这只是一个简单的示例代码,实际上一个真正的图书管理系统应该包括更多的功能和复杂性。
阅读全文
相关推荐












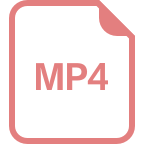