帮我用C++写一个代码实现转轮密码机的加密与解密
时间: 2023-03-26 17:03:38 浏览: 108
好的,我可以回答这个问题。以下是一个简单的转轮密码机加密和解密的 C 代码示例:
```c
#include <stdio.h>
#include <string.h>
#define ROTORS 3
#define ALPHABET_SIZE 26
void rotate_rotors(int *rotors) {
rotors[] = (rotors[] + 1) % ALPHABET_SIZE;
if (rotors[] == ) {
rotors[1] = (rotors[1] + 1) % ALPHABET_SIZE;
if (rotors[1] == ) {
rotors[2] = (rotors[2] + 1) % ALPHABET_SIZE;
}
}
}
char encrypt_char(char c, int *rotors, char **wiring) {
int index = c - 'A';
for (int i = ; i < ROTORS; i++) {
index = (index + rotors[i]) % ALPHABET_SIZE;
index = wiring[i][index] - 'A';
}
rotate_rotors(rotors);
return index + 'A';
}
char decrypt_char(char c, int *rotors, char **wiring) {
int index = c - 'A';
for (int i = ROTORS - 1; i >= ; i--) {
index = wiring[i][index] - 'A';
index = (index - rotors[i] + ALPHABET_SIZE) % ALPHABET_SIZE;
}
rotate_rotors(rotors);
return index + 'A';
}
void encrypt(char *plaintext, char *ciphertext, int *rotors, char **wiring) {
int len = strlen(plaintext);
for (int i = ; i < len; i++) {
ciphertext[i] = encrypt_char(plaintext[i], rotors, wiring);
}
ciphertext[len] = '\';
}
void decrypt(char *ciphertext, char *plaintext, int *rotors, char **wiring) {
int len = strlen(ciphertext);
for (int i = ; i < len; i++) {
plaintext[i] = decrypt_char(ciphertext[i], rotors, wiring);
}
plaintext[len] = '\';
}
int main() {
char *wiring[ROTORS] = {
"BDFHJLCPRTXVZNYEIWGAKMUSQO",
"AJDKSIRUXBLHWTMCQGZNPYFVOE",
"EKMFLGDQVZNTOWYHXUSPAIBRCJ"
};
int rotors[ROTORS] = {, , };
char plaintext[] = "HELLO";
char ciphertext[strlen(plaintext) + 1];
encrypt(plaintext, ciphertext, rotors, wiring);
printf("Ciphertext: %s\n", ciphertext);
char decrypted[strlen(ciphertext) + 1];
decrypt(ciphertext, decrypted, rotors, wiring);
printf("Decrypted: %s\n", decrypted);
return ;
}
```
这个代码实现了一个简单的转轮密码机,使用了三个转轮和三个固定的接线板。`encrypt` 函数将明文加密为密文,`decrypt` 函数将密文解密为明文。`rotate_rotors` 函数将转轮向前旋转一个位置。`encrypt_char` 和 `decrypt_char` 函数分别加密和解密一个字符。
相关推荐
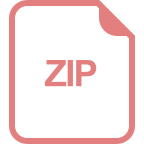
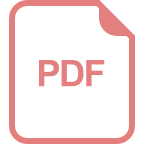














