在pcl中,crop滤波器如何设置setTranslation和setRotation
时间: 2024-03-12 16:49:35 浏览: 315
在PCL中,CropBox滤波器也可以使用setTranslation和setRotation方法来设置平移和旋转参数。
首先,需要创建一个CropBox对象,例如:
```
pcl::CropBox<pcl::PointXYZ> crop_box;
```
然后,可以使用setTranslation和setRotation方法来设置平移和旋转参数。这两个方法接受一个Eigen::Vector3f类型的参数,分别表示x、y、z方向上的平移或旋转。例如,如果想要将CropBox向右平移10个单位,则可以使用以下代码:
```
Eigen::Vector3f translation(10, 0, 0);
crop_box.setTranslation(translation);
```
同样地,如果想要将CropBox逆时针旋转45度,则可以使用以下代码:
```
Eigen::Vector3f rotation(0, 0, -45);
crop_box.setRotation(rotation);
```
需要注意的是,CropBox滤波器的平移和旋转参数是相对于滤波器自身的坐标系的,而不是全局坐标系。因此,在设置参数时需要考虑好滤波器的初始位置和方向。
相关问题
pcl 的统计滤波器的函数
PCL(Point Cloud Library)的统计滤波器是一种基于统计学原理的滤波器,可以用于去除点云中的离群点等噪声。以下是PCL中统计滤波器的函数:
1. pcl::StatisticalOutlierRemoval:统计滤波器的主要实现类,可以通过设置均值距离和标准差倍数来过滤离群点。
2. setMeanK:设置用于计算每个点的邻居点数。
3. setStddevMulThresh:设置标准差倍数的阈值。
4. setInputCloud:设置输入点云。
5. setNegative:设置是否保留离群点。
6. setSaveLeafLayout:设置是否保存叶子布局。
7. filter:执行统计滤波器。
使用示例:
```cpp
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud (new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointCloud<pcl::PointXYZ>::Ptr filtered_cloud (new pcl::PointCloud<pcl::PointXYZ>);
// 读取点云数据
pcl::io::loadPCDFile ("cloud.pcd", *cloud);
// 创建统计滤波器对象
pcl::StatisticalOutlierRemoval<pcl::PointXYZ> sor;
sor.setInputCloud (cloud);
sor.setMeanK (50);
sor.setStddevMulThresh (1.0);
// 执行统计滤波
sor.filter (*filtered_cloud);
// 保存滤波后的点云数据
pcl::io::savePCDFileBinary ("filtered_cloud.pcd", *filtered_cloud);
```
用c++实现pcl的crop filter过滤一个带有平移和旋转的矩形框点云
您好!要实现这个功能,可以按照以下步骤进行:
1. 定义一个裁剪框(矩形框),可以使用 PCL 中的 `pcl::CropBox` 进行定义,需要设置框的中心、大小和旋转角度等参数。
2. 将需要裁剪的点云加载到 PCL 中,并将其转换为 PCL 中的点云对象,例如 `pcl::PointCloud<pcl::PointXYZ>::Ptr cloud`。
3. 对点云进行裁剪,可以调用 `pcl::CropBox` 的 `setInputCloud()` 方法设置输入点云,然后调用 `filter()` 方法进行裁剪。裁剪后的点云会保存在 `pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_filtered` 对象中。
下面是示例代码:
```c++
#include <pcl/filters/crop_box.h>
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud (new pcl::PointCloud<pcl::PointXYZ>);
// 加载点云数据到 cloud 对象中
// ...
pcl::CropBox<pcl::PointXYZ> crop_box;
crop_box.setInputCloud(cloud);
// 设置裁剪框的参数,例如:
crop_box.setTranslation(Eigen::Vector3f(0.1, 0.2, 0.3));
crop_box.setRotation(Eigen::Quaternionf(0.1, 0.2, 0.3, 0.4));
crop_box.setMin(Eigen::Vector4f(-0.2, -0.3, -0.5, 1.0));
crop_box.setMax(Eigen::Vector4f(0.2, 0.3, 0.5, 1.0));
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_filtered (new pcl::PointCloud<pcl::PointXYZ>);
crop_box.filter(*cloud_filtered);
// cloud_filtered 中保存了裁剪后的点云数据
```
需要注意的是,裁剪框的参数需要根据实际情况进行设置,例如框的中心、大小和旋转角度等。同时,需要保证点云数据能够准确地匹配到裁剪框中,可以通过调整点云的位置和姿态等参数进行调整。
阅读全文
相关推荐
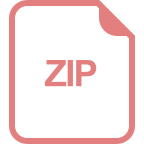
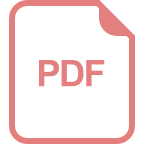
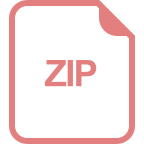
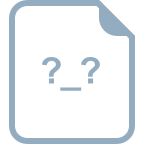
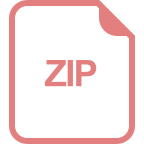
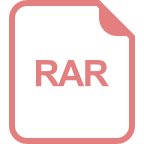
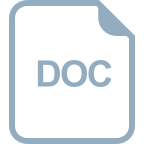


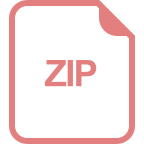