yuv数据 转base64实例
时间: 2023-12-03 17:51:22 浏览: 47
下面是将 YUV 数据转换为 Base64 编码的 Python 代码示例:
```python
import base64
# 假设 YUV 数据存储在 yuv_data 变量中
# YUV 数据可以是任何长度的字节数组,例如从文件中读取或通过网络接收
yuv_data = b'\x00\x12\x34\x56\x78\x9a\xbc\xde\xff'
# 将 YUV 数据编码为 Base64 字符串
base64_data = base64.b64encode(yuv_data).decode('utf-8')
# 输出 Base64 编码后的字符串
print(base64_data)
```
这段代码假设 YUV 数据已经存储在变量 `yuv_data` 中。首先使用 Python 标准库的 `base64.b64encode()` 函数将 YUV 数据编码为 Base64 字符串。然后使用字符串的 `decode()` 方法将字节字符串转换为普通的字符串,以便输出和保存。
相关问题
c++ yuv数据 转base64实例
下面是一个简单的c++代码示例,将YUV数据转换为Base64编码:
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <cstring>
#include <vector>
#include <algorithm>
#include "base64.h" // base64编码实现
using namespace std;
int main() {
// 读取YUV数据
ifstream in("test.yuv", ios::in | ios::binary);
if (!in) {
cerr << "Failed to open file." << endl;
return -1;
}
vector<char> yuvData((istreambuf_iterator<char>(in)), istreambuf_iterator<char>());
// 将yuvData转换为Base64编码
string base64Data = base64_encode(reinterpret_cast<const unsigned char*>(yuvData.data()), yuvData.size());
// 输出Base64编码
cout << base64Data << endl;
return 0;
}
```
需要使用第三方库进行Base64编码的实现,可以使用开源的base64.h头文件,它提供了base64_encode和base64_decode两个函数,用于编码和解码Base64数据。注意,此示例中的yuvData应该是原始YUV数据的指针,因此需要使用reinterpret_cast来转换为无符号字符指针。
c++ yuv数据 转base64数据代码
以下是将 YUV 数据转换为 Base64 编码的 C++ 代码示例:
```
#include <iostream>
#include <fstream>
#include <string>
#include <cstring>
#include <cstdlib>
#include <cmath>
using namespace std;
// 编码表
const string base64_chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/";
// 将 YUV 数据转换为 Base64 编码
string encode(const unsigned char* yuv_data, int width, int height) {
int y_size = width * height;
int uv_size = y_size / 4;
int data_size = y_size + uv_size * 2;
unsigned char* data = new unsigned char[data_size];
memcpy(data, yuv_data, y_size);
memcpy(data + y_size, yuv_data + y_size * 5 / 4, uv_size);
memcpy(data + y_size + uv_size, yuv_data + y_size, uv_size);
// 将数据按照 6 位分组,转换成 Base64 编码
string encoded_data;
for (int i = 0; i < data_size; i += 3) {
unsigned char b1 = data[i];
unsigned char b2 = i + 1 < data_size ? data[i + 1] : 0;
unsigned char b3 = i + 2 < data_size ? data[i + 2] : 0;
unsigned char c1 = b1 >> 2;
unsigned char c2 = ((b1 & 0x03) << 4) | ((b2 & 0xf0) >> 4);
unsigned char c3 = ((b2 & 0x0f) << 2) | ((b3 & 0xc0) >> 6);
unsigned char c4 = b3 & 0x3f;
encoded_data += base64_chars[c1];
encoded_data += base64_chars[c2];
encoded_data += i + 1 < data_size ? base64_chars[c3] : '=';
encoded_data += i + 2 < data_size ? base64_chars[c4] : '=';
}
delete[] data;
return encoded_data;
}
int main() {
// 假设有一张 640x480 的 YUV420P 格式的图像
int width = 640;
int height = 480;
int y_size = width * height;
int uv_size = y_size / 4;
unsigned char* yuv_data = new unsigned char[y_size + uv_size * 2];
ifstream fin("test.yuv", ios::binary);
fin.read((char*)yuv_data, y_size + uv_size * 2);
fin.close();
// 将 YUV 数据转换为 Base64 编码
string encoded_data = encode(yuv_data, width, height);
cout << "Base64 编码后的数据:" << endl << encoded_data << endl;
delete[] yuv_data;
return 0;
}
```
需要注意的是,这里假设输入的 YUV 数据为 YUV420P 格式,即先存储亮度分量 Y,再按照 UV 交错的方式存储色度分量 U 和 V。如果输入的 YUV 数据格式不同,需要根据实际情况进行修改。
相关推荐
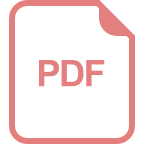
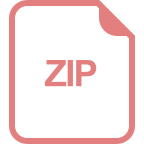












