请用Python写出如何在一张图像上绘制mask的代码
时间: 2024-05-11 20:15:32 浏览: 12
涉及技术问题,可以直接回答:
```python
import cv2
import numpy as np
# 加载图像
img = cv2.imread("test.jpg")
# 创建 mask
mask = np.zeros(img.shape[:2], dtype=np.uint8)
# 绘制 mask
cv2.circle(mask, center=(300,300), radius=200, color=255, thickness=-1)
# 将 mask 应用于图像
img_masked = cv2.bitwise_and(img, img, mask=mask)
# 显示图像和 mask
cv2.imshow("image", img)
cv2.imshow("mask", mask)
cv2.imshow("masked_image", img_masked)
cv2.waitKey(0)
```
这个代码展示了如何在一张图像上绘制一个圆形的 mask,并将其应用于图像上,最终生成一个带 mask 效果的新图像。
相关问题
请用Python与matplotlib写出如何在一张图像上绘制mask的代码
下面是使用Python与matplotlib在一张图像上绘制mask的代码:
```python
import matplotlib.pyplot as plt
import numpy as np
# 加载图像
img = plt.imread('image.png')
# 创建一个黑色的遮罩
mask = np.zeros_like(img[:,:,0])
# 绘制矩形区域的遮罩
mask[100:250, 100:300] = 1
# 将遮罩应用于原始图像
masked_img = np.copy(img)
masked_img[:,:,0] = np.where(mask==1, 255, masked_img[:,:,0])
masked_img[:,:,1] = np.where(mask==1, 0, masked_img[:,:,1])
masked_img[:,:,2] = np.where(mask==1, 0, masked_img[:,:,2])
# 绘制原始图像和遮罩后的图像
fig, axs = plt.subplots(1, 2)
axs[0].imshow(img)
axs[0].set_title('Original Image')
axs[1].imshow(masked_img)
axs[1].set_title('Masked Image')
plt.show()
```
这段代码假设你要绘制一个长宽为200x200,左上角坐标为(100, 100)的矩形区域的遮罩,并将其应用于名为"image.png"的原始图像。运行后,将在一个窗口中呈现出原始图像和遮罩后的图像。
用python写一个程序,研究雷达图像的干旱中心
A: 首先,我们需要获取雷达图像数据。这可以通过使用Python中的库来实现,其中一个比较流行的库是pyart。接下来,我们可以使用Python中的一些图像处理函数来识别干旱中心。
其中,一个简单的方法是基于颜色阈值。我们可以将图像转换为灰度图像,并应用一个阈值,使得颜色值小于该阈值的像素被视为表示干旱。使用numpy库可以快速地实现这些操作。
下面是一个示例代码:
```
import matplotlib.pyplot as plt
import numpy as np
import pyart
# 读取雷达图像数据
radar = pyart.io.read('/path/to/radar_image')
# 获取图像中的反射率数据(DBZ)
dbz = radar.fields['reflectivity']['data']
# 将DBZ转换为灰度图像
gray = (dbz - dbz.min()) / (dbz.max() - dbz.min()) * 255
gray = gray.astype(np.uint8)
# 指定干旱阈值
threshold = 150
# 二值化图像
mask = np.zeros_like(gray)
mask[gray < threshold] = 255
# 查找连通区域
label_image, num_labels = ndimage.label(mask)
# 计算干旱中心
props = measure.regionprops(label_image)
# 打印干旱中心坐标
for prop in props:
print(prop.centroid)
# 显示图像和二值化结果
plt.subplot(121)
plt.imshow(gray)
plt.subplot(122)
plt.imshow(mask, cmap='gray')
plt.show()
```
这段代码将会读取指定路径下的雷达图像,将DBZ数据转换为灰度图像,并将图像二值化,以找出符合干旱条件像素的连通区域。接着,它将使用measure库中的regionprops函数来计算每个连通区域的中心坐标,并将结果打印出来。
最后,它绘制了原图像和二值化结果的子图,以便您可以查看计算结果。
相关推荐
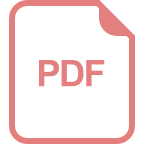












