stm32f103c8t6单总线与DS18B20温度传感器的通讯
时间: 2023-08-28 12:08:01 浏览: 129
DS18B20是一种数字温度传感器,采用单总线协议进行通讯,因此可以直接连接到STM32F103C8T6的单总线引脚上进行通讯。
下面是一个简单的示例代码,可以读取DS18B20传感器的温度值:
```c
#include "stm32f10x.h"
#define GPIO_PORT GPIOA
#define GPIO_PIN GPIO_Pin_0
void delay_us(uint32_t us)
{
uint32_t i;
for(i=0; i<us*8; i++);
}
void ds18b20_init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIO_PORT, &GPIO_InitStructure);
}
void ds18b20_write_byte(uint8_t data)
{
uint8_t i;
for(i=0; i<8; i++)
{
if((data>>i)&0x01)
GPIO_PORT->BSRR = GPIO_PIN;
else
GPIO_PORT->BRR = GPIO_PIN;
delay_us(5);
GPIO_PORT->BSRR = GPIO_PIN;
delay_us(60);
}
}
uint8_t ds18b20_read_byte(void)
{
uint8_t i, data=0;
for(i=0; i<8; i++)
{
GPIO_PORT->BRR = GPIO_PIN;
delay_us(2);
GPIO_PORT->BSRR = GPIO_PIN;
delay_us(6);
if(GPIO_ReadInputDataBit(GPIO_PORT, GPIO_PIN))
data |= 0x01<<i;
delay_us(50);
}
return data;
}
void ds18b20_convert(void)
{
ds18b20_init();
ds18b20_write_byte(0xCC); // skip ROM
ds18b20_write_byte(0x44); // convert T
}
float ds18b20_read_temp(void)
{
uint16_t temp;
float result;
ds18b20_init();
ds18b20_write_byte(0xCC); // skip ROM
ds18b20_write_byte(0xBE); // read scratchpad
temp = ds18b20_read_byte();
temp |= ds18b20_read_byte()<<8;
result = (float)temp/16.0;
return result;
}
int main(void)
{
float temp;
ds18b20_convert(); // start conversion
while(1)
{
temp = ds18b20_read_temp(); // read temperature
// do something with the temperature value
}
}
```
以上示例代码仅供参考,具体实现还需要根据实际情况进行调整。
阅读全文
相关推荐
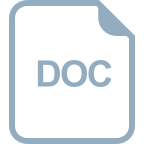
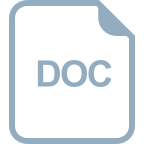
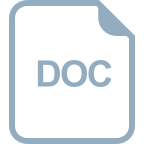

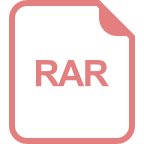
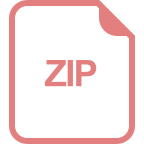
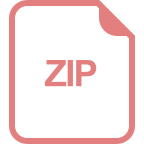
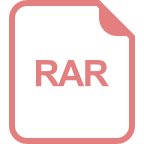



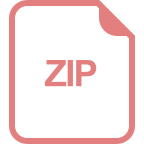
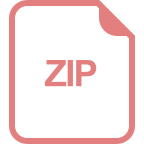
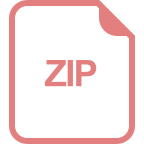
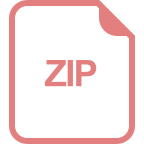
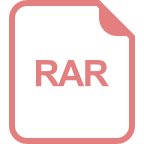
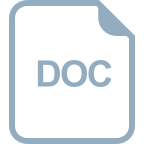
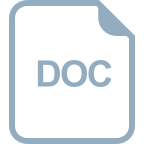
