基于VS2022写一个删除文件检索记录的程序
时间: 2023-09-14 20:02:41 浏览: 41
好的,以下是一个简单的删除文件检索记录的程序,你可以在VS2022中创建一个新的控制台应用程序项目,并将以下代码粘贴到`main.cpp`文件中,然后构建并运行该程序。
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <filesystem>
using namespace std;
namespace fs = std::filesystem;
// 记录文件路径和时间戳的结构体
struct Record {
string path;
time_t timestamp;
};
// 从文件中读取记录并返回一个记录向量
vector<Record> read_records(const string& filename) {
vector<Record> records;
ifstream infile(filename);
if (infile.is_open()) {
string line;
while (getline(infile, line)) {
// 每一行包含文件路径和时间戳,通过空格分隔
size_t space_pos = line.find(' ');
if (space_pos != string::npos) {
string path = line.substr(0, space_pos);
time_t timestamp = stoi(line.substr(space_pos + 1));
records.push_back(Record{ path, timestamp });
}
}
infile.close();
}
return records;
}
// 将记录向量写入文件
void write_records(const string& filename, const vector<Record>& records) {
ofstream outfile(filename);
if (outfile.is_open()) {
for (const auto& record : records) {
outfile << record.path << ' ' << record.timestamp << endl;
}
outfile.close();
}
}
int main() {
string record_file = "records.txt";
vector<Record> records = read_records(record_file);
// 遍历记录向量并删除已经不存在的文件
for (auto iter = records.begin(); iter != records.end();) {
if (!fs::exists(iter->path)) {
iter = records.erase(iter);
} else {
++iter;
}
}
// 将更新后的记录向量写回文件
write_records(record_file, records);
return 0;
}
```
该程序会从指定的记录文件中读取记录向量,然后遍历记录向量并检查每个记录对应的文件是否存在。如果文件不存在,则将该记录从记录向量中删除。最后,程序将更新后的记录向量写回记录文件中。
请注意,在这个简单的示例程序中,我们只检查文件是否存在,而不进行任何其他检查(例如是否可以删除文件)。在实际应用中,需要根据需要进行更复杂的检查和处理。
相关推荐
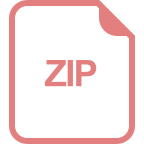
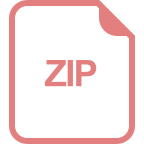
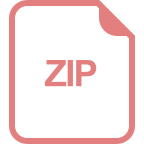














