创建考勤表 create table Attendance( worknumber char(15) not null , year varchar(10) , month varchar(10) , day varchar(10), iswork varchar(10) )character set utf8;
时间: 2024-04-02 09:31:56 浏览: 87
好的,您创建的是一个名为Attendance的表,包含五个字段:worknumber、year、month、day和iswork。其中worknumber字段为非空的字符类型,长度为15;year、month和day字段为字符类型,长度为10;iswork字段为字符类型,长度为10。表的字符集为utf8。
相关问题
CREATE TABLE EmployeeInfo ( ID INT PRIMARY KEY, Name VARCHAR(50) NOT NULL, Gender VARCHAR(10) NOT NULL, Birthday DATE, Department VARCHAR(50), Position VARCHAR(50), HireDate DATE, Salary DECIMAL(10, 2), Phone VARCHAR(20), Email VARCHAR(50) ); CREATE TABLE SalaryInfo ( ID INT PRIMARY KEY, EmployeeID INT NOT NULL, Salary DECIMAL(10, 2) NOT NULL, StartDate DATE NOT NULL, EndDate DATE, FOREIGN KEY (EmployeeID) REFERENCES EmployeeInfo(ID) ); CREATE TABLE AttendanceInfo ( ID INT PRIMARY KEY, EmployeeID INT NOT NULL, AttendanceDate DATE NOT NULL, SignTime DATETIME, LeaveTime DATETIME, FOREIGN KEY (EmployeeID) REFERENCES EmployeeInfo(ID) ); CREATE TABLE WelfareInfo ( ID INT PRIMARY KEY, EmployeeID INT NOT NULL, WelfareType VARCHAR(50) NOT NULL, WelfareAmount DECIMAL(10, 2) NOT NULL, WelfareDate DATE NOT NULL, FOREIGN KEY (EmployeeID) REFERENCES EmployeeInfo(ID) ); CREATE TABLE DepartmentInfo ( ID INT PRIMARY KEY, DepartmentName VARCHAR(50) NOT NULL, ManagerID INT, Description VARCHAR(200) );
好的,以下是向AttendanceInfo表插入五条内容的SQL语句示例:
INSERT INTO AttendanceInfo(ID, EmployeeID, AttendanceDate, SignTime, LeaveTime) VALUES (1, 1001, '2021-07-01', '2021-07-01 09:00:00', '2021-07-01 18:00:00');
INSERT INTO AttendanceInfo(ID, EmployeeID, AttendanceDate, SignTime, LeaveTime) VALUES (2, 1001, '2021-07-02', '2021-07-02 09:10:00', '2021-07-02 18:00:00');
INSERT INTO AttendanceInfo(ID, EmployeeID, AttendanceDate, SignTime, LeaveTime) VALUES (3, 1002, '2021-07-01', '2021-07-01 09:05:00', '2021-07-01 18:30:00');
INSERT INTO AttendanceInfo(ID, EmployeeID, AttendanceDate, SignTime, LeaveTime) VALUES (4, 1002, '2021-07-02', '2021-07-02 09:20:00', '2021-07-02 18:20:00');
INSERT INTO AttendanceInfo(ID, EmployeeID, AttendanceDate, SignTime, LeaveTime) VALUES (5, 1003, '2021-07-01', '2021-07-01 09:15:00', '2021-07-01 18:15:00');
注意,在实际插入数据时,你需要根据自己的表结构和具体需要进行调整和修改。
Attendance(考勤表) 字段名 类型 注释 其他 id int 序号 主键,非空 attendancename varchar(50) 考勤 空 basepay decimal(10) 基本工资 空 根据以上内容用java写出增删改查方法类
以下是一个参考实现:
```java
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class AttendanceDAO {
private Connection conn;
public AttendanceDAO(Connection conn) {
this.conn = conn;
}
public void addAttendance(Attendance attendance) throws SQLException {
String sql = "INSERT INTO Attendance (id, attendancename, basepay) VALUES (?, ?, ?)";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setInt(1, attendance.getId());
stmt.setString(2, attendance.getAttendanceName());
stmt.setBigDecimal(3, attendance.getBasePay());
stmt.executeUpdate();
}
public void updateAttendance(Attendance attendance) throws SQLException {
String sql = "UPDATE Attendance SET attendancename = ?, basepay = ? WHERE id = ?";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setString(1, attendance.getAttendanceName());
stmt.setBigDecimal(2, attendance.getBasePay());
stmt.setInt(3, attendance.getId());
stmt.executeUpdate();
}
public void deleteAttendance(int id) throws SQLException {
String sql = "DELETE FROM Attendance WHERE id = ?";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setInt(1, id);
stmt.executeUpdate();
}
public List<Attendance> getAllAttendance() throws SQLException {
String sql = "SELECT * FROM Attendance";
PreparedStatement stmt = conn.prepareStatement(sql);
ResultSet rs = stmt.executeQuery();
List<Attendance> attendances = new ArrayList<>();
while (rs.next()) {
int id = rs.getInt("id");
String attendanceName = rs.getString("attendancename");
BigDecimal basePay = rs.getBigDecimal("basepay");
Attendance attendance = new Attendance(id, attendanceName, basePay);
attendances.add(attendance);
}
return attendances;
}
public Attendance getAttendanceById(int id) throws SQLException {
String sql = "SELECT * FROM Attendance WHERE id = ?";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setInt(1, id);
ResultSet rs = stmt.executeQuery();
if (rs.next()) {
String attendanceName = rs.getString("attendancename");
BigDecimal basePay = rs.getBigDecimal("basepay");
Attendance attendance = new Attendance(id, attendanceName, basePay);
return attendance;
} else {
return null;
}
}
}
```
其中 `Attendance` 类代表一个考勤记录,有三个字段:`id`、`attendanceName`、`basePay`。`AttendanceDAO` 类是对考勤记录进行增删改查的方法类,其中 `conn` 是一个数据库连接对象。这里使用了 `PreparedStatement` 来防止 SQL 注入攻击。
阅读全文
相关推荐
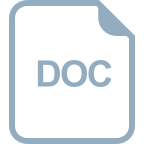
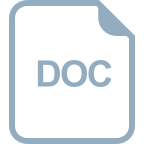
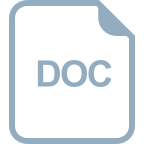
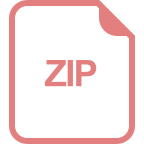
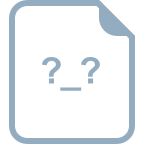
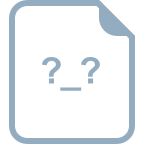
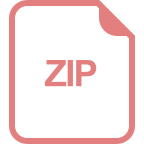
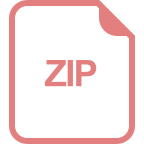
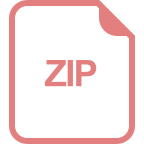
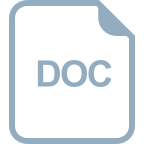






