springboot发送邮箱
时间: 2023-07-06 09:26:55 浏览: 47
好的,你可以使用 Spring Boot 集成 JavaMailSender 来发送邮件。以下是一个简单的示例:
1. 在 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
```
2. 在 application.properties 文件中添加邮件配置:
```properties
spring.mail.host=smtp.example.com
spring.mail.username=your-email@example.com
spring.mail.password=your-password
spring.mail.properties.mail.smtp.auth=true
spring.mail.properties.mail.smtp.starttls.enable=true
spring.mail.properties.mail.smtp.starttls.required=true
spring.mail.properties.mail.smtp.ssl.trust=smtp.example.com
```
3. 创建一个邮件服务类:
```java
@Service
public class EmailService {
@Autowired
private JavaMailSender mailSender;
public void sendSimpleEmail(String toAddress, String subject, String content) {
SimpleMailMessage message = new SimpleMailMessage();
message.setFrom("your-email@example.com");
message.setTo(toAddress);
message.setSubject(subject);
message.setText(content);
mailSender.send(message);
}
}
```
4. 在需要发送邮件的地方调用邮件服务类:
```java
@Autowired
private EmailService emailService;
// ...
emailService.sendSimpleEmail("to-address@example.com", "Test Email", "This is a test email.");
```
以上就是使用 Spring Boot 发送邮件的简单示例。当然,你还可以使用更高级的邮件发送方式,例如使用 HTML 格式、附件、图片等。具体实现可以参考 Spring Boot 官方文档。
相关推荐
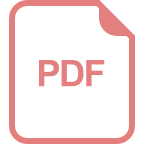
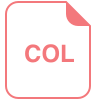
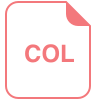
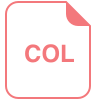
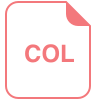
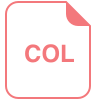








