帮我用JAVA写一个二分查找
时间: 2023-08-31 19:21:58 浏览: 47
### 回答1:
好的,这是 Java 代码实现的二分查找:
```
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5};
int target = 4;
int result = binarySearch(arr, target);
System.out.println("The target is located at index: " + result);
}
}
```
这个代码实现了一个简单的二分查找,如果找到了目标值,就返回目标值所在的索引;如果没有找到目标值,就返回 -1。
### 回答2:
当然可以帮您用JAVA编写一个二分查找算法。
二分查找,也称为折半查找,是一种在有序数组中查找指定元素的算法。它的基本思想是将待查找区间的中间位置的元素与目标元素进行比较,根据比较结果选择将待查找区间缩小为前半部分或后半部分,并重复这个过程,直到找到目标元素或待查找区间为空。
下面是使用JAVA语言编写的二分查找算法示例:
```java
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // 如果未找到目标元素,返回-1
}
public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int target = 5;
int index = binarySearch(arr, target);
if (index != -1) {
System.out.println("目标元素 " + target + " 在数组中的索引为 " + index);
} else {
System.out.println("目标元素 " + target + " 未在数组中找到");
}
}
}
```
运行上述代码,输出为“目标元素 5 在数组中的索引为 4”。
希望以上代码对您有所帮助!如果有任何问题,请随时向我提问。
### 回答3:
二分查找,也称为折半查找,是一种常用的查找算法。其基本思想是,将查找的范围逐渐缩小为一半,直到找到目标元素或确定目标元素不存在。
以下是用JAVA实现二分查找的代码示例:
```java
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (arr[mid] == target) {
return mid;
}
if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // 表示目标元素不存在
}
public static void main(String[] args) {
int[] arr = {1, 3, 5, 7, 9}; // 假设数组已经按照升序排列
int target = 5;
int index = binarySearch(arr, target);
if (index != -1) {
System.out.println("目标元素在数组中的索引位置为:" + index);
} else {
System.out.println("目标元素不存在于数组中");
}
}
}
```
以上代码定义了一个`BinarySearch`类,其中包含一个静态方法`binarySearch`用于实现二分查找。在`main`方法中,创建了一个升序排列的整型数组`arr`,指定了目标元素`target`为5。通过调用`binarySearch`方法,返回目标元素在数组中的索引位置(如果存在),否则返回-1。最后,根据返回的结果进行输出。
相关推荐
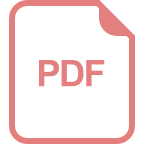
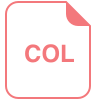
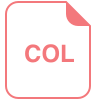

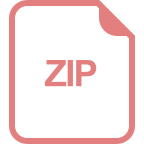
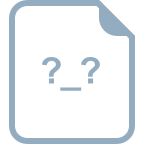
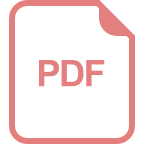
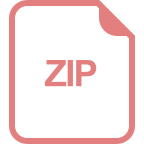