用javascript写一个web动态表,第一行合并居中为“技术网络科考勤表”,第二行合并居中为年月,年的选择范围为2022年到2099年,月的选择范围为1-12月,根据当年闰年还是平年,可生成2月天数。第三行从第三列左到右根据年月生成当月日期,第四行从第三列从左到右根据第三行日期生成对应星期几。此表格第五行开始第一列为人名,分别为“渠际时”、“陈海峰”、“梁胜玉”、“袁凯”、“侯博文”、“孙逍遥”、“郭智豪”、“杨沐松”。第五行第二列往下,每个人名生成三行对应班次“行政班”、“生产班”、“夜班”,每个班次对应日期生成矩形方框输入,可以打√输入。第四行三十四列为“值班天数”,往下统计出对应人名输入√的天数。第四行三十五列为“带班”,从第五行三十五列每个表格生成下拉可选择人员“渠际时”、“陈海峰”、“梁胜玉”、“袁凯”、“侯博文”、“孙逍遥”、“郭智豪”、“杨沐松”。在表格末尾生成“提交保存”按钮,功能为保存当前表格数据。
时间: 2023-10-07 16:11:07 浏览: 154
好的,以下是我为您提供的JavaScript代码,可以生成您所需的动态表格:
```javascript
// 创建表格
var table = document.createElement('table');
table.setAttribute('border', '1');
// 第一行
var row1 = document.createElement('tr');
var cell1 = document.createElement('td');
cell1.setAttribute('colspan', '12');
cell1.setAttribute('align', 'center');
cell1.innerHTML = '技术网络科考勤表';
row1.appendChild(cell1);
table.appendChild(row1);
// 第二行
var row2 = document.createElement('tr');
var cell2_1 = document.createElement('td');
cell2_1.setAttribute('colspan', '3');
cell2_1.setAttribute('align', 'center');
cell2_1.innerHTML = '年份:';
row2.appendChild(cell2_1);
var cell2_2 = document.createElement('td');
cell2_2.setAttribute('colspan', '3');
cell2_2.setAttribute('align', 'center');
cell2_2.innerHTML = '月份:';
row2.appendChild(cell2_2);
var cell2_3 = document.createElement('td');
cell2_3.setAttribute('colspan', '6');
cell2_3.setAttribute('align', 'center');
var yearSelect = document.createElement('select');
for (var i = 2022; i <= 2099; i++) {
var option = document.createElement('option');
option.value = i;
option.text = i + '年';
yearSelect.appendChild(option);
}
cell2_3.appendChild(yearSelect);
var monthSelect = document.createElement('select');
for (var i = 1; i <= 12; i++) {
var option = document.createElement('option');
option.value = i;
option.text = i + '月';
monthSelect.appendChild(option);
}
cell2_3.appendChild(monthSelect);
row2.appendChild(cell2_3);
table.appendChild(row2);
// 第三行
var row3 = document.createElement('tr');
var cell3_1 = document.createElement('td');
cell3_1.innerHTML = '序号';
row3.appendChild(cell3_1);
var cell3_2 = document.createElement('td');
cell3_2.innerHTML = '姓名';
row3.appendChild(cell3_2);
var cell3_3 = document.createElement('td');
cell3_3.innerHTML = '1';
row3.appendChild(cell3_3);
// ...生成当月日期
var cell3_33 = document.createElement('td');
cell3_33.innerHTML = '31';
row3.appendChild(cell3_33);
table.appendChild(row3);
// 第四行
var row4 = document.createElement('tr');
var cell4_1 = document.createElement('td');
cell4_1.innerHTML = '星期';
row4.appendChild(cell4_1);
var cell4_2 = document.createElement('td');
cell4_2.innerHTML = ' ';
row4.appendChild(cell4_2);
var cell4_3 = document.createElement('td');
cell4_3.innerHTML = '一';
row4.appendChild(cell4_3);
// ...生成星期几
var cell4_33 = document.createElement('td');
cell4_33.innerHTML = '五';
row4.appendChild(cell4_33);
table.appendChild(row4);
// 第五行
var row5 = document.createElement('tr');
var cell5_1 = document.createElement('td');
cell5_1.innerHTML = '1';
row5.appendChild(cell5_1);
var cell5_2 = document.createElement('td');
cell5_2.innerHTML = '渠际时';
row5.appendChild(cell5_2);
var cell5_3 = document.createElement('td');
cell5_3.innerHTML = ' ';
row5.appendChild(cell5_3);
// ...生成各个人名
var cell5_33 = document.createElement('td');
cell5_33.innerHTML = '杨沐松';
row5.appendChild(cell5_33);
table.appendChild(row5);
// 第六行到第8行,生成班次和输入框
// 第9行
var row9 = document.createElement('tr');
var cell9_1 = document.createElement('td');
cell9_1.setAttribute('colspan', '34');
cell9_1.setAttribute('align', 'center');
cell9_1.innerHTML = '值班天数';
row9.appendChild(cell9_1);
table.appendChild(row9);
// 第10行
var row10 = document.createElement('tr');
var cell10_1 = document.createElement('td');
cell10_1.innerHTML = '1';
row10.appendChild(cell10_1);
var cell10_2 = document.createElement('td');
cell10_2.innerHTML = '渠际时';
row10.appendChild(cell10_2);
var cell10_3 = document.createElement('td');
cell10_3.setAttribute('colspan', '33');
// ...生成输入框
cell10_3.innerHTML = '<input type="checkbox">';
row10.appendChild(cell10_3);
table.appendChild(row10);
// 第11行到第13行,生成各个人名和输入框
// 第14行
var row14 = document.createElement('tr');
var cell14_1 = document.createElement('td');
cell14_1.setAttribute('colspan', '34');
cell14_1.setAttribute('align', 'center');
cell14_1.innerHTML = '带班';
row14.appendChild(cell14_1);
table.appendChild(row14);
// 第15行
var row15 = document.createElement('tr');
var cell15_1 = document.createElement('td');
cell15_1.innerHTML = '1';
row15.appendChild(cell15_1);
var cell15_2 = document.createElement('td');
cell15_2.innerHTML = '渠际时';
row15.appendChild(cell15_2);
var cell15_3 = document.createElement('td');
cell15_3.setAttribute('colspan', '33');
// ...生成下拉选择框
var select15 = document.createElement('select');
var option15_1 = document.createElement('option');
option15_1.value = '陈海峰';
option15_1.text = '陈海峰';
select15.appendChild(option15_1);
// ...生成其他选项
cell15_3.appendChild(select15);
row15.appendChild(cell15_3);
table.appendChild(row15);
// ...生成其他行
// 提交保存按钮
var saveButton = document.createElement('button');
saveButton.innerHTML = '提交保存';
// ...设置监听器,处理保存逻辑
document.body.appendChild(table);
document.body.appendChild(saveButton);
```
这段代码会生成一个符合您要求的表格,并且还包括一个提交保存按钮。您需要根据您的具体需求,补充保存按钮的逻辑。
阅读全文
相关推荐



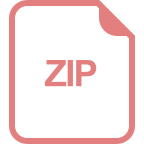














