使用java写一个阿里云oss上传
时间: 2024-06-12 08:05:12 浏览: 13
以下是使用Java代码实现阿里云OSS上传的示例:
1. 添加依赖
```
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-sdk-oss</artifactId>
<version>3.13.1</version>
</dependency>
```
2. 初始化OSSClient对象
```
String endpoint = "yourEndpoint";
String accessKeyId = "yourAccessKeyId";
String accessKeySecret = "yourAccessKeySecret";
OSS ossClient = new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
```
3. 创建Bucket
```
String bucketName = "yourBucketName";
if (!ossClient.doesBucketExist(bucketName)) {
ossClient.createBucket(bucketName);
}
```
4. 上传文件
```
String objectName = "yourObjectName";
String filePath = "yourFilePath";
File file = new File(filePath);
ossClient.putObject(bucketName, objectName, file);
```
5. 关闭OSSClient对象
```
ossClient.shutdown();
```
完整的示例代码如下:
```
import com.aliyun.oss.OSS;
import com.aliyun.oss.OSSClientBuilder;
import java.io.File;
public class OssUploadDemo {
public static void main(String[] args) {
String endpoint = "yourEndpoint";
String accessKeyId = "yourAccessKeyId";
String accessKeySecret = "yourAccessKeySecret";
String bucketName = "yourBucketName";
String objectName = "yourObjectName";
String filePath = "yourFilePath";
OSS ossClient = new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
if (!ossClient.doesBucketExist(bucketName)) {
ossClient.createBucket(bucketName);
}
File file = new File(filePath);
ossClient.putObject(bucketName, objectName, file);
ossClient.shutdown();
}
}
```
相关推荐
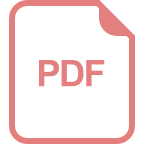
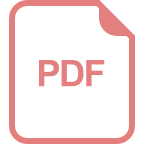
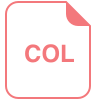













