迟入网时隙编号设计 代码 Omnet
时间: 2024-03-17 16:40:34 浏览: 33
对于迟入网时隙编号设计,可以通过Omnet++进行模拟实现。具体步骤如下:
1. 创建Omnet++工程并添加必要的库文件。
2. 在工程中创建一个名为"slot"的模块,用于模拟时隙的传输和接收。
3. 在"slot"模块中定义时隙的编号和长度,并定义时隙传输的相关参数,如传输速率、信道状态等。
4. 在"slot"模块中实现时隙传输和接收的逻辑,包括时隙编号的生成和识别、数据的传输和接收等。
5. 在"slot"模块中添加统计功能,用于记录时隙传输的成功率、传输延迟等指标。
6. 在工程中创建其他模块,如发送方、接收方等,用于模拟实际网络环境下的数据传输。
7. 在发送方模块中实现数据的生成和传输,包括选择合适的时隙进行传输等。
8. 在接收方模块中实现数据的接收和处理,包括识别正确的时隙进行接收等。
9. 运行模拟程序并记录相关指标,进行分析和优化。
代码实现如下(仅供参考):
```
// slot模块.h文件
#ifndef __SLOT_H_
#define __SLOT_H_
#include <omnetpp.h>
using namespace omnetpp;
class Slot : public cSimpleModule
{
private:
int slotNum; // 时隙编号
int slotLength; // 时隙长度
double transmitRate; // 传输速率
bool channelStatus; // 信道状态
protected:
virtual void initialize();
virtual void handleMessage(cMessage *msg);
public:
void generateSlotNum(); // 生成时隙编号
int getSlotNum(); // 获取时隙编号
int getSlotLength(); // 获取时隙长度
double getTransmitRate(); // 获取传输速率
bool getChannelStatus(); // 获取信道状态
void setChannelStatus(bool status); // 设置信道状态
};
#endif
// slot模块.cc文件
#include "slot.h"
void Slot::initialize()
{
slotNum = 0;
slotLength = 10;
transmitRate = 1.0;
channelStatus = true;
generateSlotNum();
}
void Slot::handleMessage(cMessage *msg)
{
delete msg;
}
void Slot::generateSlotNum()
{
slotNum++;
if (slotNum > 100)
slotNum = 1;
}
int Slot::getSlotNum()
{
return slotNum;
}
int Slot::getSlotLength()
{
return slotLength;
}
double Slot::getTransmitRate()
{
return transmitRate;
}
bool Slot::getChannelStatus()
{
return channelStatus;
}
void Slot::setChannelStatus(bool status)
{
channelStatus = status;
}
// 发送方模块.cc文件
#include <omnetpp.h>
#include "slot.h"
using namespace omnetpp;
class Sender : public cSimpleModule
{
private:
Slot *slot;
cMessage *msg;
protected:
virtual void initialize();
virtual void handleMessage(cMessage *msg);
};
Define_Module(Sender);
void Sender::initialize()
{
slot = getParentModule()->getSubmodule("slot");
// 创建数据包
msg = new cMessage("data");
msg->setByteLength(100);
scheduleAt(0, msg);
}
void Sender::handleMessage(cMessage *msg)
{
if (msg == this->msg)
{
// 选择合适的时隙进行传输
if (slot->getChannelStatus())
{
int slotNum = slot->getSlotNum();
int slotLength = slot->getSlotLength();
double transmitRate = slot->getTransmitRate();
double transmitTime = (double)slotLength / transmitRate;
EV << "Send data in slot " << slotNum << endl;
// 发送数据包
sendDelayed(msg, transmitTime, "out");
// 更新时隙状态
slot->setChannelStatus(false);
scheduleAt(simTime() + transmitTime, new cMessage("channel_idle"));
}
else
{
EV << "Channel is busy, wait for next slot" << endl;
scheduleAt(simTime() + slot->getSlotLength(), msg);
}
}
else
{
// 时隙空闲,更新状态
slot->setChannelStatus(true);
delete msg;
}
}
// 接收方模块.cc文件
#include <omnetpp.h>
#include "slot.h"
using namespace omnetpp;
class Receiver : public cSimpleModule
{
private:
Slot *slot;
protected:
virtual void initialize();
virtual void handleMessage(cMessage *msg);
};
Define_Module(Receiver);
void Receiver::initialize()
{
slot = getParentModule()->getSubmodule("slot");
}
void Receiver::handleMessage(cMessage *msg)
{
// 识别正确的时隙进行接收
int slotNum = slot->getSlotNum();
if (strcmp(msg->getName(), "data") == 0 && slotNum == msg->getArrivalGate()->getIndex())
{
EV << "Receive data in slot " << slotNum << endl;
}
delete msg;
}
```
以上代码仅为示例,具体实现需根据实际需求进行调整和完善。
相关推荐
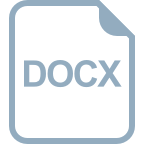














