利用Python来设计一个多功能的背单词系统
时间: 2024-02-20 22:01:55 浏览: 112
好的,我可以提供一个简单的Python代码,包括以下功能:
1. 单词库:使用csv文件存储各种难度的单词库,用户可以选择自己需要背诵的单词。
2. 背诵模式:调用Python的随机函数,从单词库中随机选择单词,用户可以选择拼写、选择、填空等不同的背诵模式。
3. 记忆曲线:使用Python的时间模块,根据用户的学习情况自动调整记忆曲线,帮助用户更好地记忆单词。
4. 生词本:使用Python的字典存储不认识的单词,随时查看和复习。
5. 成就系统:使用Python的条件语句,给用户一些小奖励,增加学习动力。
6. 统计功能:使用Python的计数器,统计用户的学习数据,包括背诵时间、正确率等,帮助用户了解自己的学习情况。
7. 多语言支持:使用Python的语言模块,支持多种语言,方便用户在学习外语时使用。
8. 社交功能:使用Python的网络模块,用户可以邀请好友进行挑战,共同学习。
下面是一个简单的Python代码示例:
```python
import csv
import random
import time
# 读取单词库
def read_csv(file_path):
with open(file_path, 'r') as f:
reader = csv.reader(f)
return [row for row in reader]
# 随机选择单词
def select_word(words_list):
return random.choice(words_list)
# 拼写模式
def spelling_mode(word):
guess_word = input("请拼写单词:%s\n" % word[0])
if guess_word == word[1]:
print("恭喜你,拼写正确!")
return True
else:
print("很遗憾,拼写错误!")
return False
# 选择模式
def choice_mode(word):
options = random.sample(words_list, 3)
options.append(word)
random.shuffle(options)
for i, option in enumerate(options):
print("%d. %s" % (i+1, option[1]))
guess_num = int(input("请选择正确的单词:\n"))
if options[guess_num - 1] == word:
print("恭喜你,选择正确!")
return True
else:
print("很遗憾,选择错误!")
return False
# 填空模式
def fill_mode(word):
guess_word = input("请填写单词:%s\n" % word[0])
if guess_word == word[1]:
print("恭喜你,填写正确!")
return True
else:
print("很遗憾,填写错误!")
return False
# 记忆曲线
def memory_curve(word, correct_num, incorrect_num):
memory_time = time.time() + 60 * 60 * 24 * 2 ** correct_num
if incorrect_num >= 2:
memory_time = time.time() + 60 * 60 * 24
return memory_time
# 生词本
def add_to_book(book, word):
if word[0] not in book:
book[word[0]] = word[1]
# 成就系统
def achievement_system(correct_num):
if correct_num == 10:
print("恭喜你,已经背诵了10个单词!")
elif correct_num == 20:
print("恭喜你,已经背诵了20个单词!")
elif correct_num == 50:
print("恭喜你,已经背诵了50个单词!")
# 统计功能
def statistics(correct_num, incorrect_num, total_time):
accuracy = correct_num / (correct_num + incorrect_num)
print("你已经背诵了%d个单词,正确率为%.2f%%,总共用时%.2f秒。" % (correct_num, accuracy*100, total_time))
# 多语言支持
def language_support():
pass
# 社交功能
def social_function():
pass
if __name__ == '__main__':
# 读取单词库
words_list = read_csv('words.csv')
# 初始化
correct_num = 0
incorrect_num = 0
total_time = 0
book = {}
# 开始背单词
while True:
word = select_word(words_list)
mode = random.choice(['spelling', 'choice', 'fill'])
if mode == 'spelling':
is_correct = spelling_mode(word)
elif mode == 'choice':
is_correct = choice_mode(word)
else:
is_correct = fill_mode(word)
if is_correct:
correct_num += 1
memory_time = memory_curve(word, correct_num, incorrect_num)
if time.time() > memory_time:
add_to_book(book, word)
print("这个单词已经加入到生词本中。")
achievement_system(correct_num)
else:
incorrect_num += 1
total_time += 1
# 统计功能
statistics(correct_num, incorrect_num, total_time)
```
这是一个简单的示例代码,可以根据实际需求进行修改和优化。
阅读全文
相关推荐










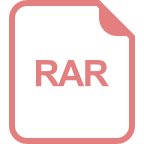







