编写一个函数,接收传入的字符串,统计大写字母的个数、小写字母的个数、数字的个数、其它字符的个数,并以元组的方式返回这些数,然后调用该函数。
时间: 2024-05-14 21:16:39 浏览: 57
好的,您可以调用以下函数来完成您的要求:
```python
def count_chars(s):
upper_count = 0
lower_count = 0
digit_count = 0
other_count = 0
for char in s:
if char.isupper():
upper_count += 1
elif char.islower():
lower_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
return (upper_count, lower_count, digit_count, other_count)
result = count_chars("Hello World 123!")
print(result)
```
这个函数接收一个字符串作为输入,并使用一个循环来遍历字符串中的每个字符。对于每个字符,使用条件语句来检查它是大写字母、小写字母、数字还是其他字符,并将对应的计数器加1。
最后,函数返回一个包含四个计数器值的元组。在这个例子中,当您传入的字符串为"Hello World 123!"时,应该会输出(2, 8, 3, 3)。
相关问题
使用Python语言编写函数,统计字符串中大写字母的个数、小写字母的个数、数字的个数、其他字符的个数,并输出。
在Python中,你可以创建一个函数来统计字符串中各类型字符的数量。这里是一个简单的示例:
```python
def count_chars(s):
uppercase_count = sum(1 for c in s if c.isupper())
lowercase_count = sum(1 for c in s if c.islower())
digit_count = sum(1 for c in s if c.isdigit())
other_count = len(s) - (uppercase_count + lowercase_count + digit_count)
print(f"大写字母个数: {uppercase_count}")
print(f"小写字母个数: {lowercase_count}")
print(f"数字个数: {digit_count}")
print(f"其他字符个数: {other_count}")
# 调用函数并传入一个字符串
count_chars("HelloWorld123!")
```
这个函数通过`isupper()`检查是否为大写字母,`islower()`检查是否为小写字母,`isdigit()`检查是否为数字。其余字符则通过计算总长度减去已知类型的字符数量得到。
编写生成器函数,接收一个字符串,分别统计大写字母、小写字母、数字、其他字符的个数,以元组的形式返回结果, 返回的元组的第一个元素依次是字符串 '大写字母'、'小写字母'、'数字' 和 '其他字符',第二个元素是相应的字符个数;
好的,下面是实现代码,注意要将字符串转化为列表,便于遍历和判断:
```python
def count_chars(s):
upper = 0
lower = 0
digit = 0
other = 0
for c in list(s):
if c.isupper():
upper += 1
elif c.islower():
lower += 1
elif c.isdigit():
digit += 1
else:
other += 1
return ('大写字母', upper), ('小写字母', lower), ('数字', digit), ('其他字符', other)
```
你可以将这段代码复制到你的 Python 环境中运行,接着调用 `count_chars` 函数并传入一个字符串,就能得到一个包含统计结果的元组了。
阅读全文
相关推荐
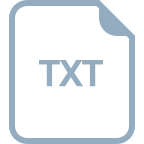













