用c++实现银行家算法
时间: 2023-06-29 15:04:38 浏览: 45
银行家算法是一种用于避免系统资源死锁的算法,以下是一个简单的 C++ 实现:
```c++
#include <iostream>
#include <vector>
using namespace std;
const int MAX_PROCESS = 100;
const int MAX_RESOURCE = 100;
int available[MAX_RESOURCE];
int allocation[MAX_PROCESS][MAX_RESOURCE];
int max_need[MAX_PROCESS][MAX_RESOURCE];
int need[MAX_PROCESS][MAX_RESOURCE];
bool finish[MAX_PROCESS];
int num_process, num_resource;
bool is_safe() {
vector<int> work(available, available + num_resource);
vector<bool> finished(num_process, false);
bool changed = true;
while (changed) {
changed = false;
for (int i = 0; i < num_process; i++) {
if (!finished[i]) {
bool possible = true;
for (int j = 0; j < num_resource; j++) {
if (need[i][j] > work[j]) {
possible = false;
break;
}
}
if (possible) {
finished[i] = true;
changed = true;
for (int j = 0; j < num_resource; j++) {
work[j] += allocation[i][j];
}
}
}
}
}
for (int i = 0; i < num_process; i++) {
if (!finished[i]) {
return false;
}
}
return true;
}
int main() {
cout << "Enter the number of processes: ";
cin >> num_process;
cout << "Enter the number of resources: ";
cin >> num_resource;
cout << "Enter the available resources: ";
for (int i = 0; i < num_resource; i++) {
cin >> available[i];
}
cout << "Enter the allocation matrix:\n";
for (int i = 0; i < num_process; i++) {
for (int j = 0; j < num_resource; j++) {
cin >> allocation[i][j];
}
}
cout << "Enter the maximum need matrix:\n";
for (int i = 0; i < num_process; i++) {
for (int j = 0; j < num_resource; j++) {
cin >> max_need[i][j];
need[i][j] = max_need[i][j] - allocation[i][j];
}
}
if (is_safe()) {
cout << "The system is in a safe state.\n";
} else {
cout << "The system is not in a safe state.\n";
}
return 0;
}
```
在代码中,`available` 数组表示当前可用的资源数量,`allocation` 数组表示每个进程已经分配的资源,`max_need` 数组表示每个进程最多需要的资源,`need` 数组表示每个进程还需要的资源数量,`finish` 数组表示每个进程是否已经执行完毕。`is_safe` 函数用于判断系统是否处于安全状态,如果是则返回 true,否则返回 false。
在程序中,首先需要输入进程数、资源数、可用资源数量、分配矩阵和最大需求矩阵,然后调用 `is_safe` 函数进行判断。如果系统处于安全状态,则输出 "The system is in a safe state.",否则输出 "The system is not in a safe state."
相关推荐
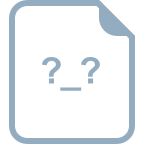
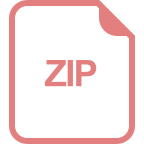













