c++代码实现ntfs文件系统文件索引遍历
时间: 2024-01-09 16:03:45 浏览: 26
由于NTFS文件系统的文件索引结构比较复杂,需要使用Windows API来实现文件索引的遍历。以下是一个示例代码,可以遍历指定目录下的所有文件和子目录。
```
#include <Windows.h>
#include <iostream>
using namespace std;
void traverseDirectory(wstring path) {
wstring searchPath = path + L"\\*.*";
WIN32_FIND_DATA fd;
HANDLE hFind = FindFirstFile(searchPath.c_str(), &fd);
if (hFind == INVALID_HANDLE_VALUE) {
return;
}
do {
wstring filename(fd.cFileName);
if (filename == L"." || filename == L"..") {
continue;
}
wstring filepath = path + L"\\" + filename;
if (fd.dwFileAttributes & FILE_ATTRIBUTE_DIRECTORY) {
// 如果是目录,递归遍历子目录
traverseDirectory(filepath);
} else {
// 如果是文件,输出文件名
wcout << filepath << endl;
}
} while (FindNextFile(hFind, &fd) != 0);
FindClose(hFind);
}
int wmain(int argc, wchar_t* argv[]) {
if (argc < 2) {
wcout << L"Usage: ntfs_traverse.exe <path>" << endl;
return 1;
}
wstring path(argv[1]);
traverseDirectory(path);
return 0;
}
```
在代码中,使用了`FindFirstFile`和`FindNextFile`函数来获取文件和目录的信息,`FILE_ATTRIBUTE_DIRECTORY`标志用来判断是否为目录。如果是目录,则递归遍历子目录,否则输出文件名。需要注意的是,由于NTFS文件系统支持Unicode字符集,因此使用了`wstring`类型来表示文件路径和文件名。
相关推荐
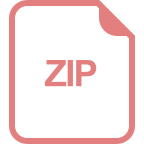
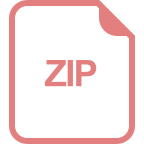
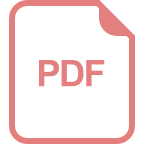














