winform里,如何读取文本文件
时间: 2023-07-18 15:20:46 浏览: 47
在WinForm中,可以通过以下代码来读取文本文件:
```csharp
string filePath = @"C:\MyFolder\MyFile.txt"; // 文本文件路径
string fileContent;
// 读取文本文件内容
try
{
// 打开文本文件
using (StreamReader sr = new StreamReader(filePath))
{
// 读取文本文件内容
fileContent = sr.ReadToEnd();
}
}
catch (Exception ex)
{
// 处理异常
fileContent = "读取文件失败:" + ex.Message;
}
// 显示文本文件内容
textBox1.Text = fileContent;
```
代码中,首先定义了一个文本文件路径`filePath`,然后通过`StreamReader`类打开文本文件并读取文件内容,将其保存到`fileContent`变量中。如果读取文件失败,则在`catch`块中处理异常并将错误信息保存到`fileContent`变量中。最后,将`fileContent`变量中的文本文件内容显示在WinForm中的文本框`textBox1`中。
需要注意的是,在使用`StreamReader`读取文本文件时,应该使用`using`语句来确保文件流对象在使用完毕后被正确地释放。此外,还需要对文件路径进行验证,确保路径有效。
相关问题
winform文本文件
您想了解如何在Winform中读取和写入文本文件吗?以下是一些基本的示例代码:
读取文本文件:
```csharp
string filePath = "C:\\example.txt";
string content = File.ReadAllText(filePath);
textBox1.Text = content;
```
在此示例中,我们使用`File.ReadAllText()`方法读取指定路径的文本文件,并将其内容存储在字符串变量中。然后,我们将该内容显示在Winform中的文本框中。
写入文本文件:
```csharp
string filePath = "C:\\example.txt";
string content = textBox1.Text;
File.WriteAllText(filePath, content);
```
在此示例中,我们使用`File.WriteAllText()`方法将Winform中文本框的内容写入指定路径的文本文件中。
请注意,这些示例代码仅适用于读取和写入纯文本文件。如果您需要读取或写入其他类型的文件(例如二进制文件),则需要使用不同的方法。
winform读取本地文件
要在 WinForms 中读取本地文件,可以使用 .NET Framework 提供的 System.IO 命名空间中的类。下面是一个简单的示例代码,可以读取文本文件并将内容显示在 TextBox 控件中:
```csharp
using System;
using System.IO;
using System.Windows.Forms;
namespace WinFormsFileReader
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog openFileDialog1 = new OpenFileDialog();
if (openFileDialog1.ShowDialog() == DialogResult.OK)
{
try
{
richTextBox1.Text = File.ReadAllText(openFileDialog1.FileName);
}
catch (Exception ex)
{
MessageBox.Show("Error: Could not read file from disk. Original error: " + ex.Message);
}
}
}
}
}
```
在上面的代码中,我们使用 OpenFileDialog 控件来打开文件对话框,然后使用 File.ReadAllText 方法读取选定的文件的内容,并将其显示在 RichTextBox 控件中。需要注意的是,我们需要在代码中添加对 System.IO 命名空间的引用,以便使用 File 类。
相关推荐
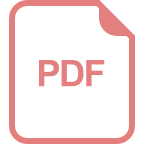
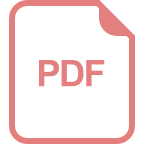












