在stm32f103c8t6中,用USART串口发送数据给wt588d时,怎么写发送串口数据的代码呢,例如在串口USART1输入:0x08 0x01 或者别的指令。如何实现播放第一首歌曲,暂停第二首歌,加大音量等等功能呢,请详细列出代码
时间: 2023-12-07 20:02:13 浏览: 76
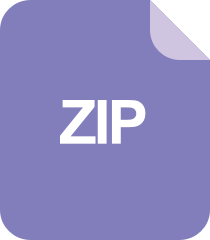
基于HAL库的stm32f103单片机实现DMA+串口的接收、发送数据功能代码
在stm32f103c8t6中,使用USART1串口发送数据给WT588D,可以采用以下步骤:
1. 配置USART1串口的GPIO口和波特率等参数;
2. 编写发送串口数据的函数,将需要发送的指令按照WT588D的协议进行拼接,并通过USART1发送出去。
以下是一个例子:
```c
#include "stm32f10x.h"
#include <stdio.h>
void USART1_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// 打开GPIOA和USART1的时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
// 配置USART1的引脚PA9和PA10
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1的工作参数
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 使能USART1
USART_Cmd(USART1, ENABLE);
}
void USART1_SendByte(uint8_t byte)
{
// 等待发送缓冲区为空
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
// 发送一个字节
USART_SendData(USART1, byte);
// 等待发送完成
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET);
}
void WT588D_PlaySong(uint8_t song_num)
{
// 指令格式:0x7E 0x02 0x01 song_num 0xEF
USART1_SendByte(0x7E); // 发送起始字节
USART1_SendByte(0x02); // 发送指令码
USART1_SendByte(0x01); // 发送数据长度
USART1_SendByte(song_num); // 发送歌曲编号
USART1_SendByte(0xEF); // 发送结束字节
}
void WT588D_PauseSong(void)
{
// 指令格式:0x7E 0x02 0x03 0x00 0xEF
USART1_SendByte(0x7E); // 发送起始字节
USART1_SendByte(0x02); // 发送指令码
USART1_SendByte(0x03); // 发送数据长度
USART1_SendByte(0x00); // 发送参数(暂停命令)
USART1_SendByte(0xEF); // 发送结束字节
}
void WT588D_IncreaseVolume(void)
{
// 指令格式:0x7E 0x02 0x04 0x00 0xEF
USART1_SendByte(0x7E); // 发送起始字节
USART1_SendByte(0x02); // 发送指令码
USART1_SendByte(0x04); // 发送数据长度
USART1_SendByte(0x00); // 发送参数(增加音量命令)
USART1_SendByte(0xEF); // 发送结束字节
}
int main(void)
{
USART1_Configuration(); // 配置USART1串口
// 播放第一首歌曲
WT588D_PlaySong(1);
// 暂停第二首歌曲
WT588D_PauseSong();
// 增加音量
WT588D_IncreaseVolume();
while (1)
{
// 循环等待
}
}
```
需要注意的是,WT588D的通信协议可以参考其数据手册,其中包括了指令格式、数据长度、参数等信息。在编写发送串口数据的函数时,需要按照协议规定的格式进行数据拼接和发送。
阅读全文
相关推荐
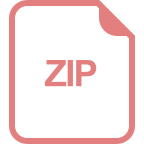
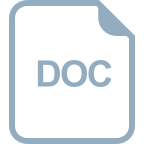
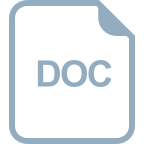







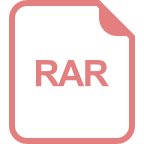
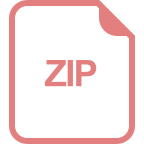
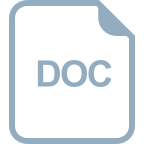


