springboot实现成功调用天气预报
时间: 2023-11-08 13:34:58 浏览: 44
1.首先需要申请获取天气预报的API接口,可以通过官方网站进行申请。
2.在Spring Boot项目中,可以通过引入相关的HTTP客户端库,如Apache HttpClient或Spring RestTemplate来实现调用API接口。
3.通过配置相关的请求参数和请求头,发送HTTP请求获取天气预报数据。
4.使用JSON解析库,如Jackson或Gson,对获取的JSON格式数据进行解析,提取需要的天气信息,并进行展示。
5.可以根据具体需求,定时或手动触发更新天气预报数据,保证数据的准确性和及时性。
相关问题
springboot用HttpClient调用天气预报接口
以下是使用HttpClient调用天气预报接口的Spring Boot示例:
1. 添加HttpClient和Jackson依赖
```xml
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</dependency>
```
2. 创建HttpClient实例
```java
@Configuration
public class HttpClientConfig {
@Bean
public CloseableHttpClient httpClient() {
return HttpClients.createDefault();
}
@Bean
public HttpClientService httpClientService(CloseableHttpClient httpClient) {
return new HttpClientService(httpClient);
}
}
```
3. 创建HttpClientService类
```java
public class HttpClientService {
private final CloseableHttpClient httpClient;
public HttpClientService(CloseableHttpClient httpClient) {
this.httpClient = httpClient;
}
public String get(String url) throws IOException {
HttpGet httpGet = new HttpGet(url);
CloseableHttpResponse response = httpClient.execute(httpGet);
try {
HttpEntity entity = response.getEntity();
if (entity != null) {
return EntityUtils.toString(entity);
}
} finally {
response.close();
}
return null;
}
}
```
4. 创建Weather类
```java
public class Weather {
private String date;
private String week;
private String weather;
private String temp;
private String humidity;
private String wind;
// getter and setter
}
```
5. 创建WeatherService类
```java
@Service
public class WeatherService {
private final HttpClientService httpClientService;
public WeatherService(HttpClientService httpClientService) {
this.httpClientService = httpClientService;
}
public List<Weather> getWeather(String city) throws IOException {
String url = "http://wthrcdn.etouch.cn/weather_mini?city=" + city;
String json = httpClientService.get(url);
ObjectMapper mapper = new ObjectMapper();
JsonNode rootNode = mapper.readTree(json);
JsonNode dataNode = rootNode.path("data");
List<Weather> weatherList = new ArrayList<>();
for (JsonNode node : dataNode.path("forecast")) {
Weather weather = mapper.treeToValue(node, Weather.class);
weatherList.add(weather);
}
return weatherList;
}
}
```
6. 测试WeatherService
```java
@RestController
public class WeatherController {
private final WeatherService weatherService;
public WeatherController(WeatherService weatherService) {
this.weatherService = weatherService;
}
@GetMapping("/weather")
public List<Weather> getWeather(@RequestParam String city) throws IOException {
return weatherService.getWeather(city);
}
}
```
现在,你可以通过访问http://localhost:8080/weather?city=北京来获取北京的天气预报信息。
springboot利用FeignClient调用天气预报接口代码
以下是使用Spring Boot和FeignClient调用天气预报接口的示例代码:
首先,在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
```
然后,在应用程序主类上添加@EnableFeignClients注解,启用FeignClient:
```java
@SpringBootApplication
@EnableFeignClients
public class WeatherApplication {
public static void main(String[] args) {
SpringApplication.run(WeatherApplication.class, args);
}
}
```
接下来,创建一个FeignClient接口来定义调用天气预报API的方法:
```java
@FeignClient(name = "weather-api", url = "https://api.weather.com")
public interface WeatherApiClient {
@GetMapping("/forecast")
ResponseEntity<String> getForecast(@RequestParam("city") String city, @RequestParam("apiKey") String apiKey);
}
```
在这个接口上,使用了FeignClient注解来指定要调用的API的名称和URL。然后,定义了一个getForecast方法,它使用@GetMapping注解来指定调用API的HTTP方法和路径,并使用@RequestParam注解来指定必需的查询参数。这个方法返回一个ResponseEntity<String>,其中包含API响应的主体。
最后,在需要调用天气预报API的代码中,注入WeatherApiClient接口,并调用getForecast方法:
```java
@RestController
public class WeatherController {
@Autowired
private WeatherApiClient weatherApiClient;
@GetMapping("/weather")
public ResponseEntity<String> getWeather(@RequestParam("city") String city, @RequestParam("apiKey") String apiKey) {
return weatherApiClient.getForecast(city, apiKey);
}
}
```
在这个控制器中,注入了WeatherApiClient接口,并在getWeather方法中调用了getForecast方法来获取天气预报数据。这个方法返回了一个ResponseEntity<String>,它包含了API响应的主体。
相关推荐
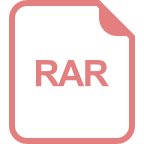
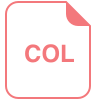
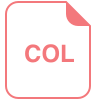
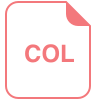
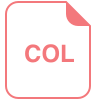
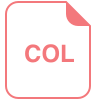







